Creating interactive user interfaces with React is an exciting and challenging task. A central concept in React is the communication between components, especially between child and parent components. In this scenario, callback functions play a vital role in sending data and events from child to parent components. In this tutorial, you will learn how to effectively implement this communication method to create reactive applications.
Key Insights
- Callback functions are essential for the communication between child and parent components in React.
- Props are used to pass callback methods from the parent to the child component.
- It is crucial to use unique keys in lists to avoid warnings and improve performance.
Step-by-step Guide
1. Create the basic structure of components
Begin by creating two main components: the parent component (e.g., App.jsx) and the child component (e.g., ToDoInput.jsx). The parent component manages the state of the application and will provide the callback function needed by the child component to transmit data.
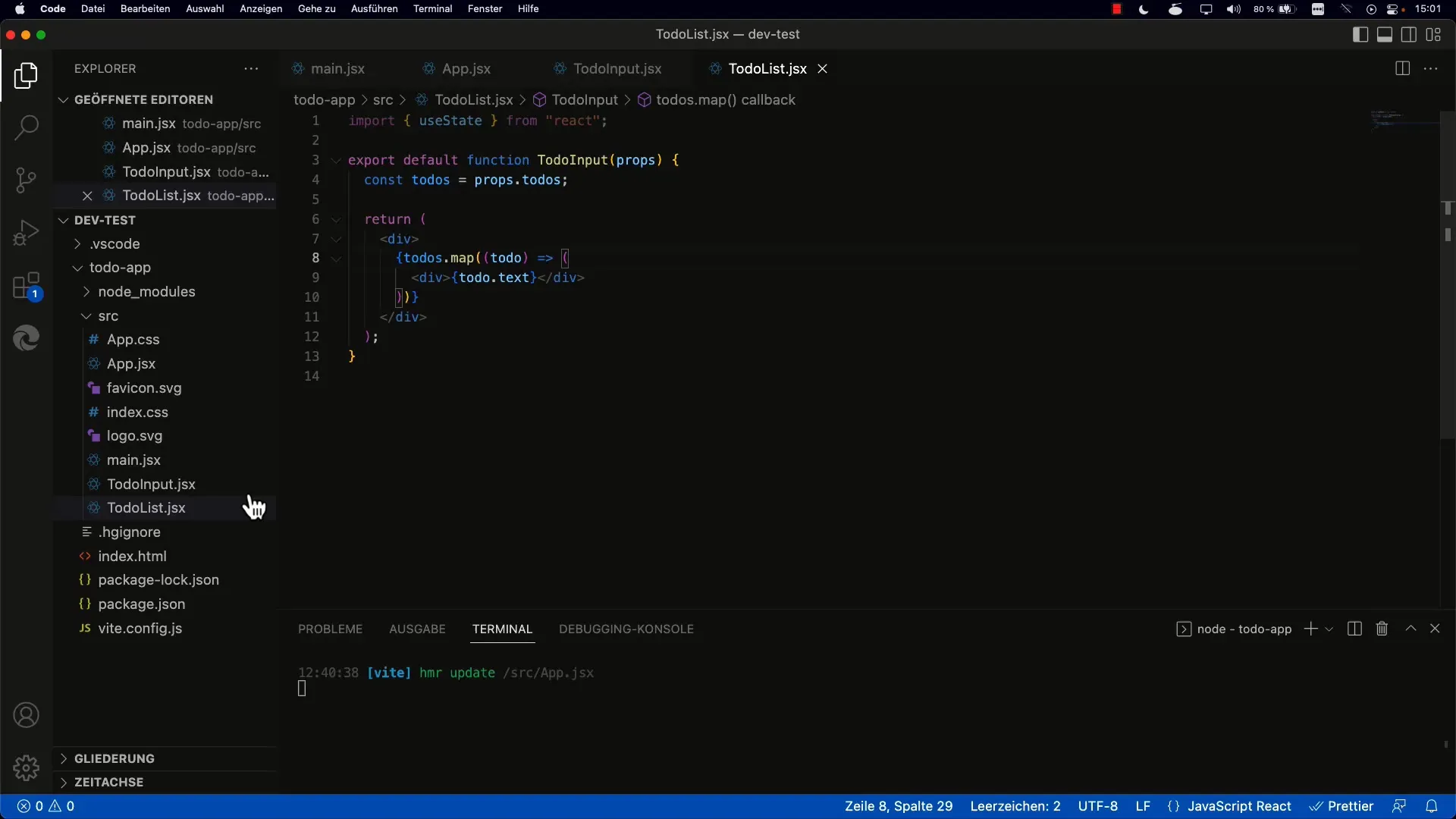
2. Create the Input component
In your child component ToDoInput.jsx, create a text input field and a button. The button should be triggered when the user wants to add a new entry. Since the button needs to interact with a callback function of the parent component, add a prop for the callback.
3. Define the Callback function
In the parent component App.jsx, you define the callback function that receives the new entry and updates the state of the ToDos. Then you need to pass this function to the child component through props.
4. Using the Callback in the child component
The child component ToDoInput.jsx now receives the callback function as a prop. Inside this component, you can work with the user input. When the user clicks the "Add" button, you call the passed callback function and pass the text entered in the input field.
5. Saving State in the parent component
When the user adds a new entry, the callback function is called, adding the new entry to the existing list of ToDos. It is essential to retain the current state and add the new element without losing the previous elements.
6. Rendering the list
After adding the new entry to the state, the list in the parent component is re-rendered. Ensure that the list of ToDos is correctly displayed in the user interface and the new entry appears.
7. Avoiding warnings during rendering
To prevent React from issuing warnings during rendering, assign a unique key to each element in a list. It is particularly important to implement this in the method that renders the ToDos.
8. Testing the application
Ensure that your application works as expected. Add multiple entries and check if they are correctly displayed in the list. This demonstrates that the communication between the components works smoothly.
9. Code optimization
To further enhance the app, consider implementing logic to create unique IDs for ToDo elements. This will help optimize performance and avoid the warning that occurs when React does not find unique keys.
10. Extending functionality
Building upon this foundation, you can expand the app by adding more features, such as deleting and checking off tasks. This will make the app even more user-friendly and functional.
Summary
In this tutorial, you have learned how to establish an effective communication between child and parent components in React. By using callback functions and props, you can create a reactive and dynamic user interface that responds to user interactions. Always remember to use unique keys in lists to optimize the performance of the application.
Frequently Asked Questions
How do I pass a callback function from a parent to a child component?You pass the callback function as a prop to the child component.
Why is it important to use unique keys in a list?Unique keys help React efficiently track elements and optimize rendering.
How can I update the state in the parent component?You can update the state using the setState method and pass the new value as a parameter, typically using a callback function.