You want to handle the styling of React components in a modern and powerful way? With the library Emotion, you can integrate CSS directly into your React components and effectively design your user interface. In this guide, I will explain the basics of styling with Emotion, how to install and apply the library, and provide you with some useful tips.
Key Insights
- Emotion allows you to write CSS in JavaScript and offers intuitive handling.
- With Emotion, you can use various styling techniques, including Template Literals and the use of the css prop.
- Style management becomes more modular with the use of Emotion, improving reusability and readability.
Step-by-Step Guide
1. Install Emotion
First, you need to install Emotion in your project. Open the terminal and navigate to your project directory. Here, run the following command to install the Emotion library:
npm install @emotion/react @emotion/styled
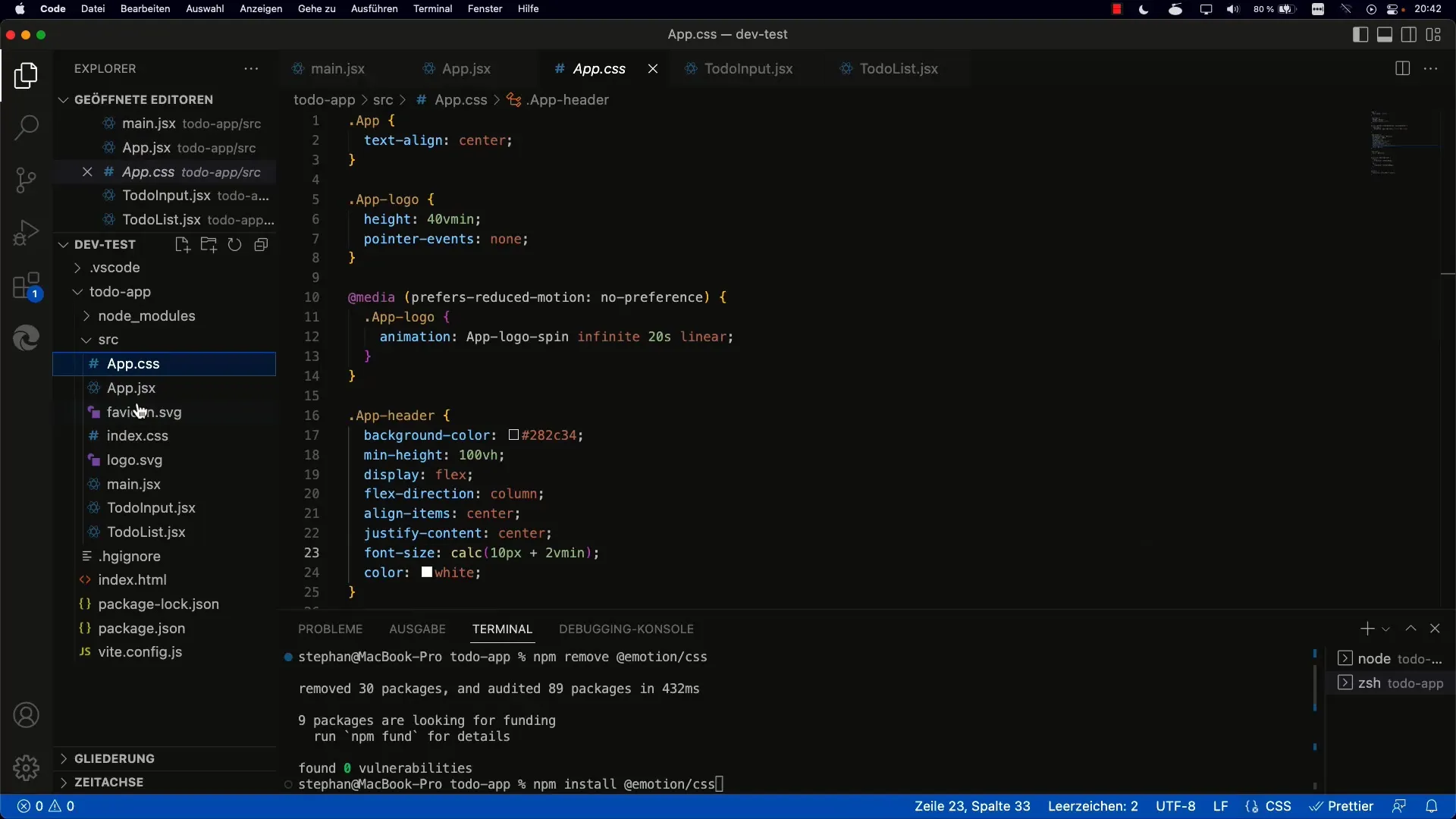
2. Import CSS
In your App.jsx file, you can use Emotion. You should import Emotion to use the css function and also import the styled element. Add the following to the beginning of your file:
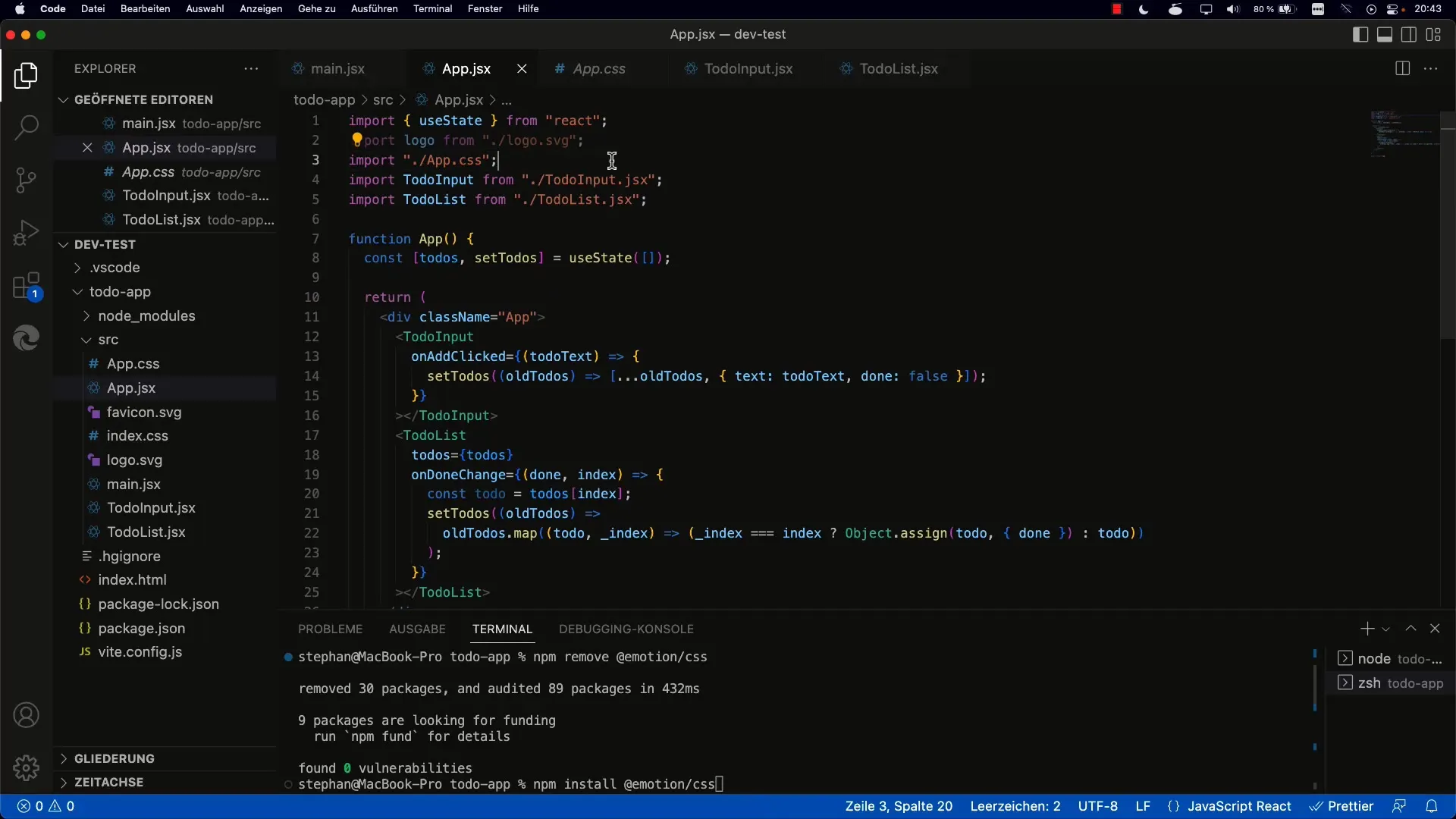
3. Styling with Template Literals
Use Emotion's css function to create a CSS variable. Define CSS conventions using Template Literals. Here's an example of how you can write a wrapper class for your app:
This code utilizes flexbox to ensure a layout arrangement.
4. Applying the Style
To apply the defined style, pass the appStyle variable in the className prop of your main HTML element. Here's how it should look:
5. Using Styled-Components
In addition to using the css variable, you can also use Styled-Components with Emotion. To create a Styled Component, you can use the following code:
This makes Container a new CSS-based component with all the defined styles.
6. Adding Media Queries
Emotion makes it easy to integrate media queries in your CSS. Simply add them to the Template Literal:
This media query changes the font size of the text when the display width is less than 600 pixels.
7. Applying Hover Effects
You can also easily define hover effects in your CSS templates:
This CSS rule changes the background color of the element when hovered over with the mouse.
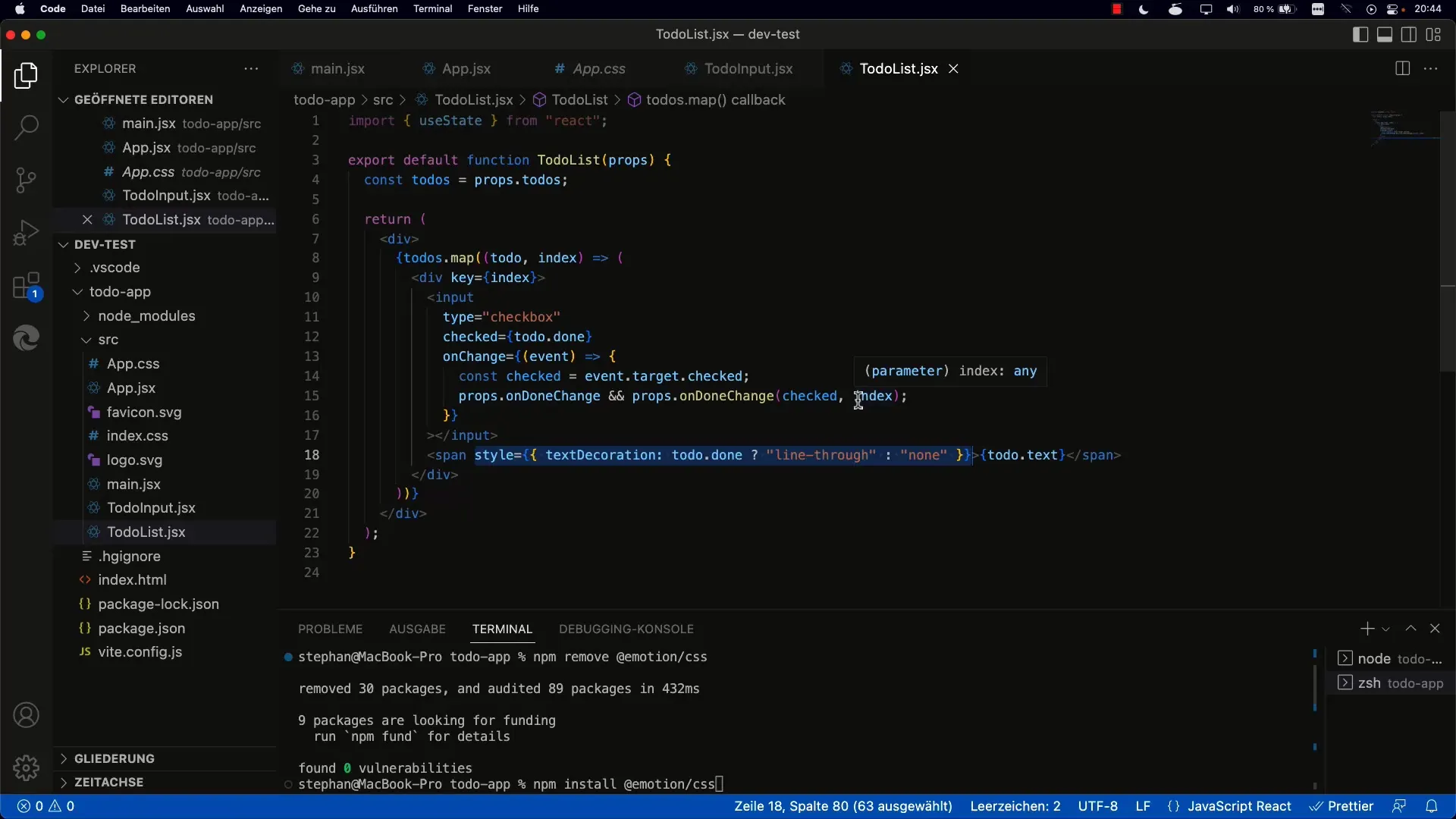
8. Combining More Complex Styles
Emotion allows you to combine multiple class names. This is enabled with the cx function:
const primaryStyle = css color: blue;;
const secondaryStyle = css color: red;;
In this case, the text is displayed in both blue and red.
9. Future Extensions
If you are managing a large project in the future, it can be helpful to organize your styles in different modules. For example, you can create commonStyles.js and export your basic styles there:
Now you can insert flexCenter into any other component.
10. Tests and Adjustments
Load your application in the browser and check if the styles are displayed correctly. Experiment with different styles and view the results in real-time.
Summary
With a combination of Emotion and React, it is possible to create realistic UI layouts. Emotion allows defining and managing CSS directly in JavaScript effectively. In this way, you can benefit from the advantages of screen density and tight integration between logic and styling.
Frequently Asked Questions
What is Emotion?Emotion is a library that enables using CSS in JavaScript and providing extensive styling options for React components.
How do I install Emotion?Use the command npm install @emotion/react @emotion/styled in your project directory.
Can I use Media Queries with Emotion?Yes, you can use Media Queries in your Emotion Styled components by writing them directly in the template literals.
Does Emotion support Hover Effects?Yes, Emotion makes it easy to add hover effects by simply defining a &:hover rule in your CSS template.