You want to create a To-Do App that also saves and loads its data even after the page is reloaded? This is not as complicated as it might sound with React and the Local Storage API. In this guide, I will show you how to persistently save the To-Dos in your browser so that they survive the session. This way, you can manage your To-Dos at any time without losing them.
Key Takeaways
- Use Local Storage to store data in the browser.
- Load the saved To-Dos at the start of the application.
- Update the Local Storage when there are changes to the To-Dos.
Step-by-Step Guide
1. Project Preparation
First, you need to ensure that you have a basic React app with a fundamental structure. If you are still struggling with the setup, you should do this beforehand.
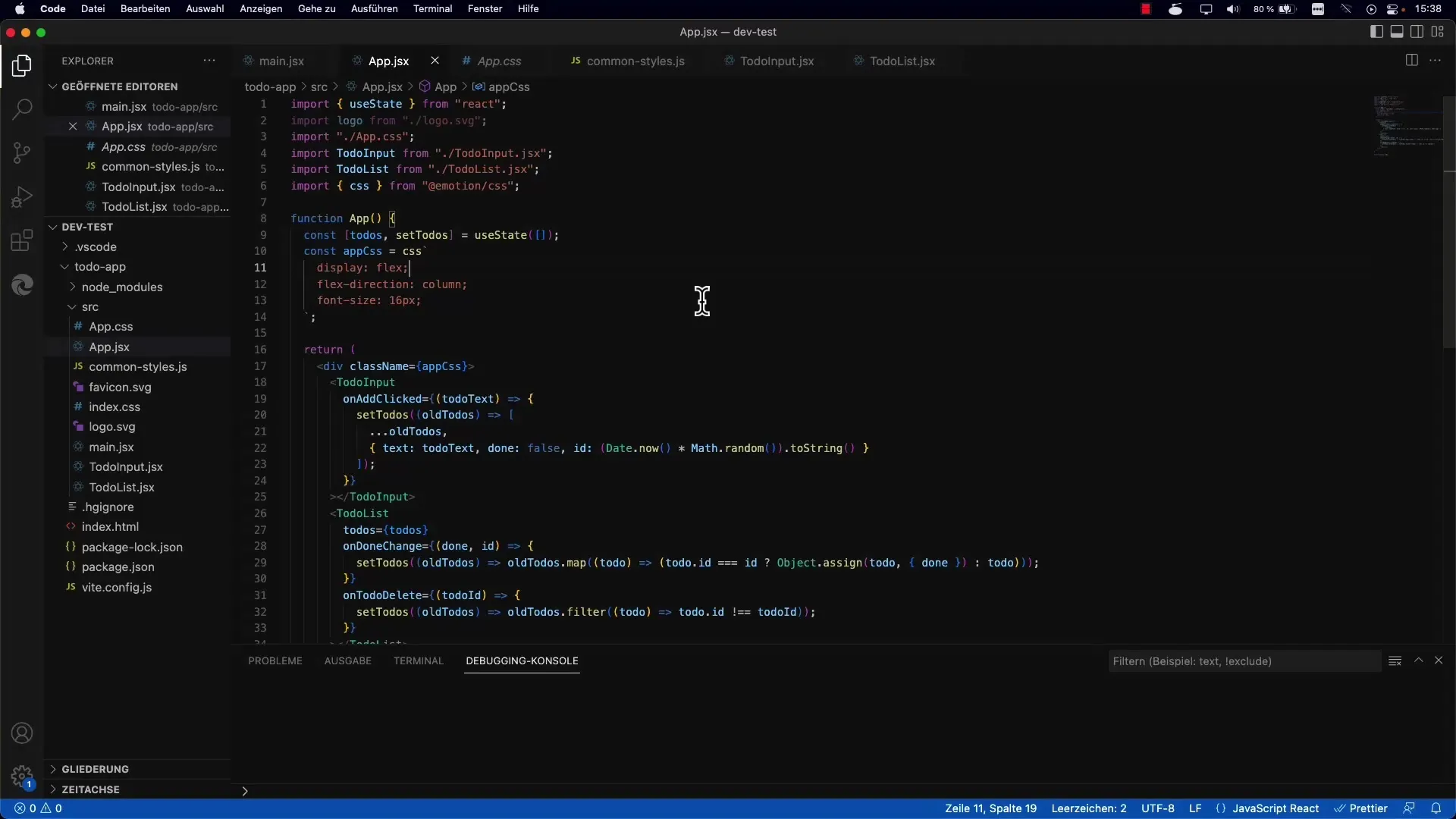
2. Defining the State Properties
Within your App.jsx file, you manage the state of your To-Dos. Here, you define state for your To-Dos, initially initialized with an empty array.
3. Loading To-Dos from Local Storage
Now you want to load the To-Dos from Local Storage instead of always starting from an empty array. To do this, you need to add a function that fetches the data at the beginning of the application.
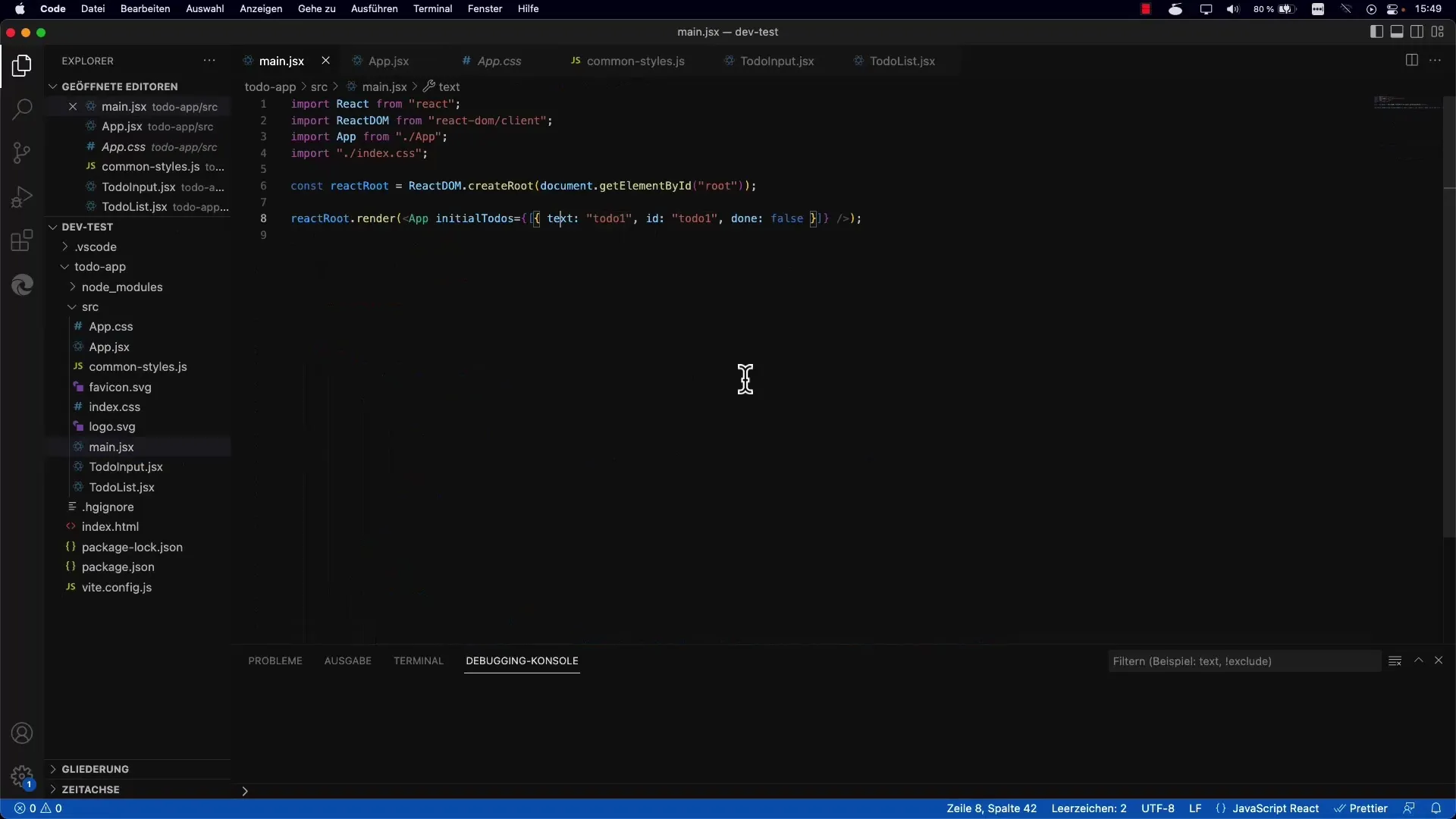
4. Implementing the load Function
You need to create a function load that fetches your To-Dos at the start. This function will retrieve the data using window.localStorage.getItem. Note that the data is stored as a JSON string and needs to be parsed into a JavaScript array using JSON.parse.
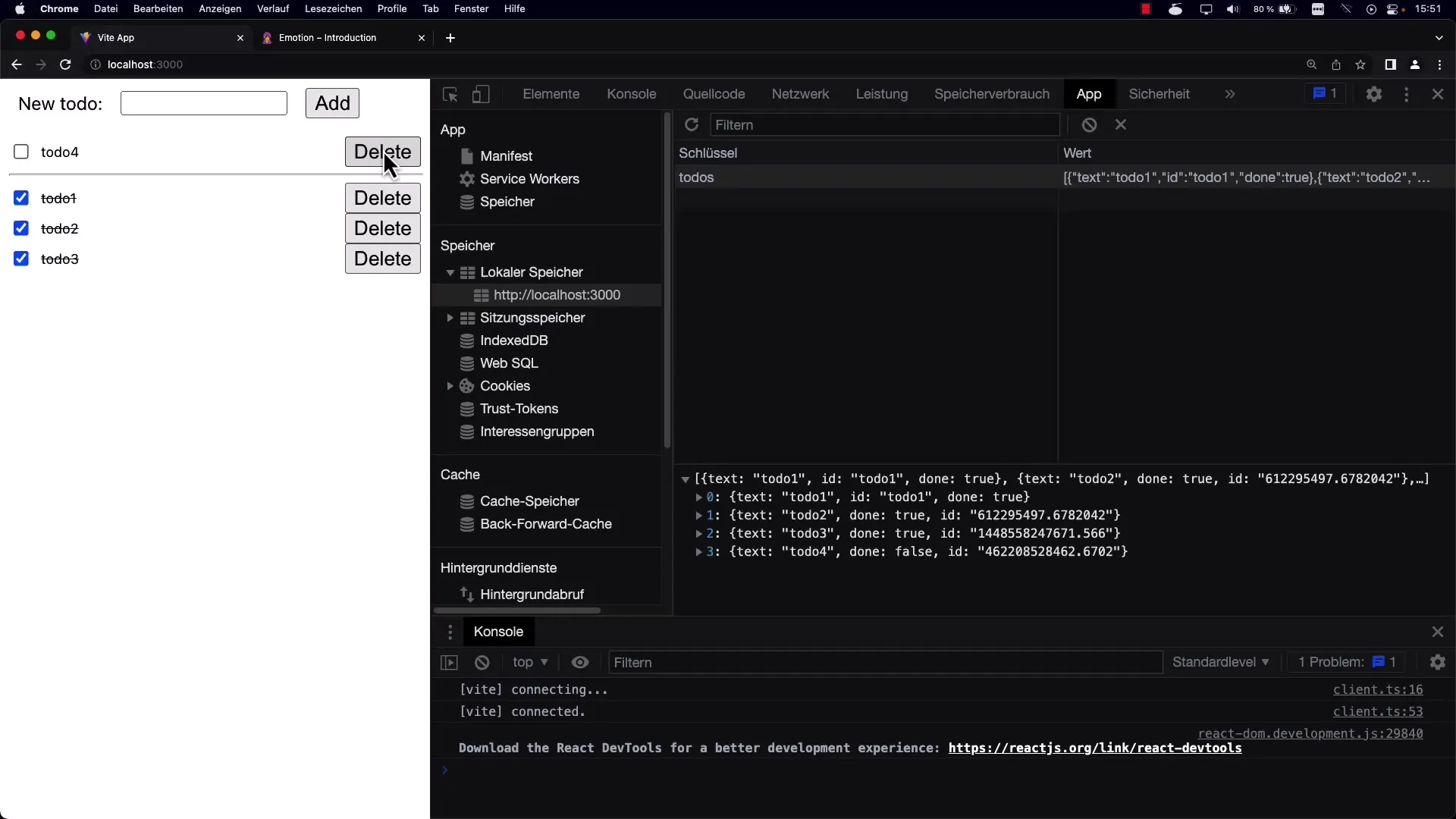
5. Saving To-Dos in Local Storage
When you add new To-Dos or edit existing ones, you must ensure that these changes are also reflected in the Local Storage. For this, you create a function save, which is called accordingly when the state is updated. You save the data under a specific key using window.localStorage.setItem.
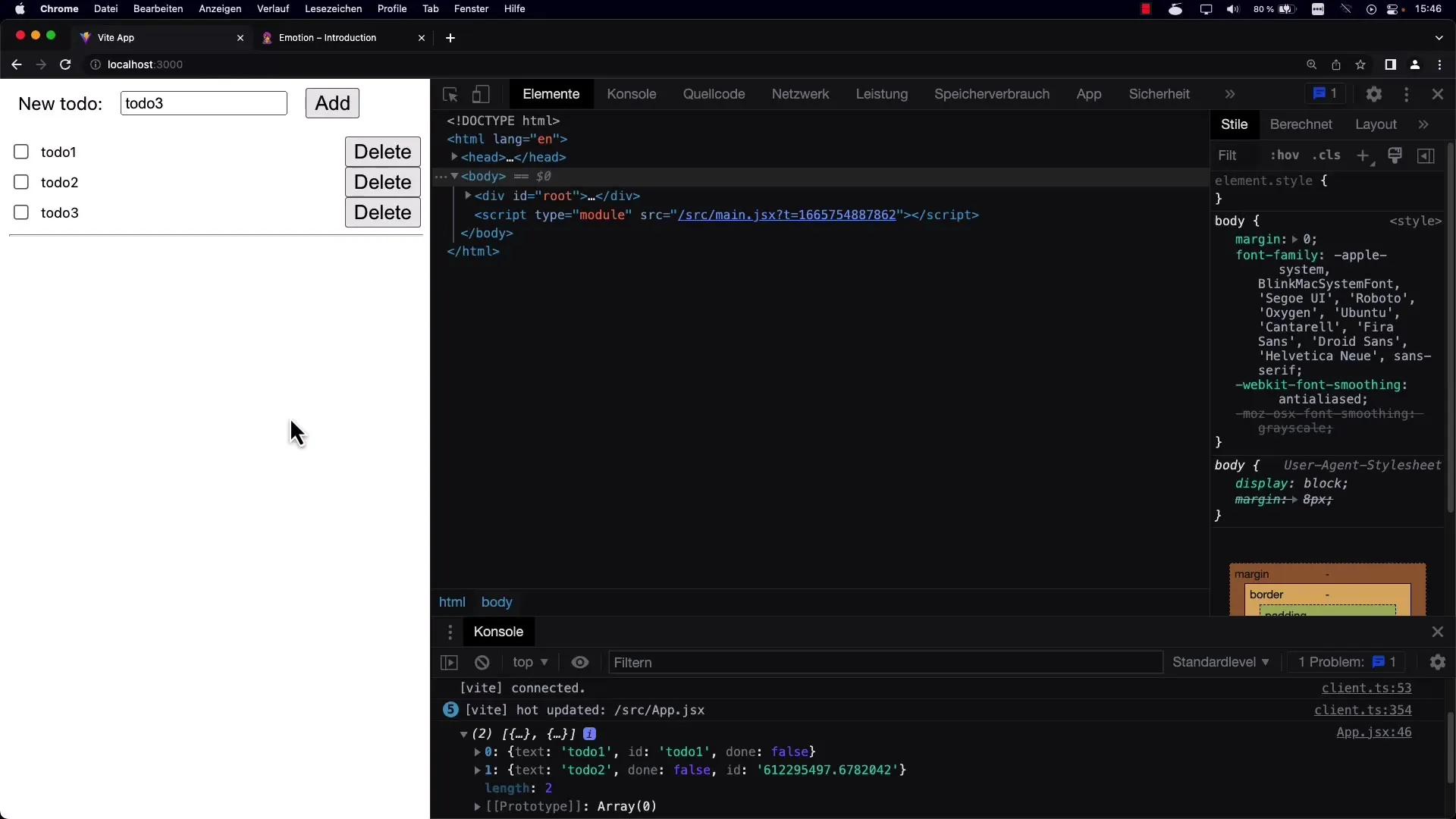
6. Testing the Saving Process
Add multiple To-Dos and then check if they are saved in Local Storage. You can do this either by visually inspecting the debugger or using your browser's developer tools.
7. Deleting To-Dos
Deleting To-Dos should also update the Local Storage. Make sure to call the save function after deleting a To-Do to ensure that the Local Storage stores the latest state.
8. Adapting the load Function for Empty Data
If the Local Storage is empty when loading the data, you want to ensure that your state is initialized with an empty array to avoid errors. Therefore, check if the data exists before loading it.
9. Creating the User Interface
Now you should ensure that your UI correctly displays all elements and that users can add new To-Dos, edit existing ones, and delete them. Verify that each action updates the UI and the Local Storage accordingly.
10. Checking the Implementation
Thoroughly test your app. Reload the page, add or remove To-Dos, and make sure everything works as expected. The data should be displayed as intended after reloading the page.
Summary
You've now learned how to create a To-Do app that stores its entries in Local Storage and reloads them when the page is reloaded. Local storage is a simple and effective way to persist data on the client side. By cleverly using localStorage, you can make your app more user-friendly and provide a better user experience.