Dealing with DOM elements in React can initially seem challenging, especially when it comes to controlling video elements. In this tutorial, we will focus on how you can control the video element in your React app using useRef. You will learn how to get references to DOM elements and effectively use them to create custom controls for your videos.
Key Takeaways
- With useRef, you can create and manage direct references to DOM elements in React.
- useEffect helps you respond to changes in components and perform actions after rendering.
- You can utilize user interactions to control the playback of video elements, especially concerning audio.
Step-by-Step Guide
1. Adding a Video Element to the App
To use the video element in your app, start by implementing a
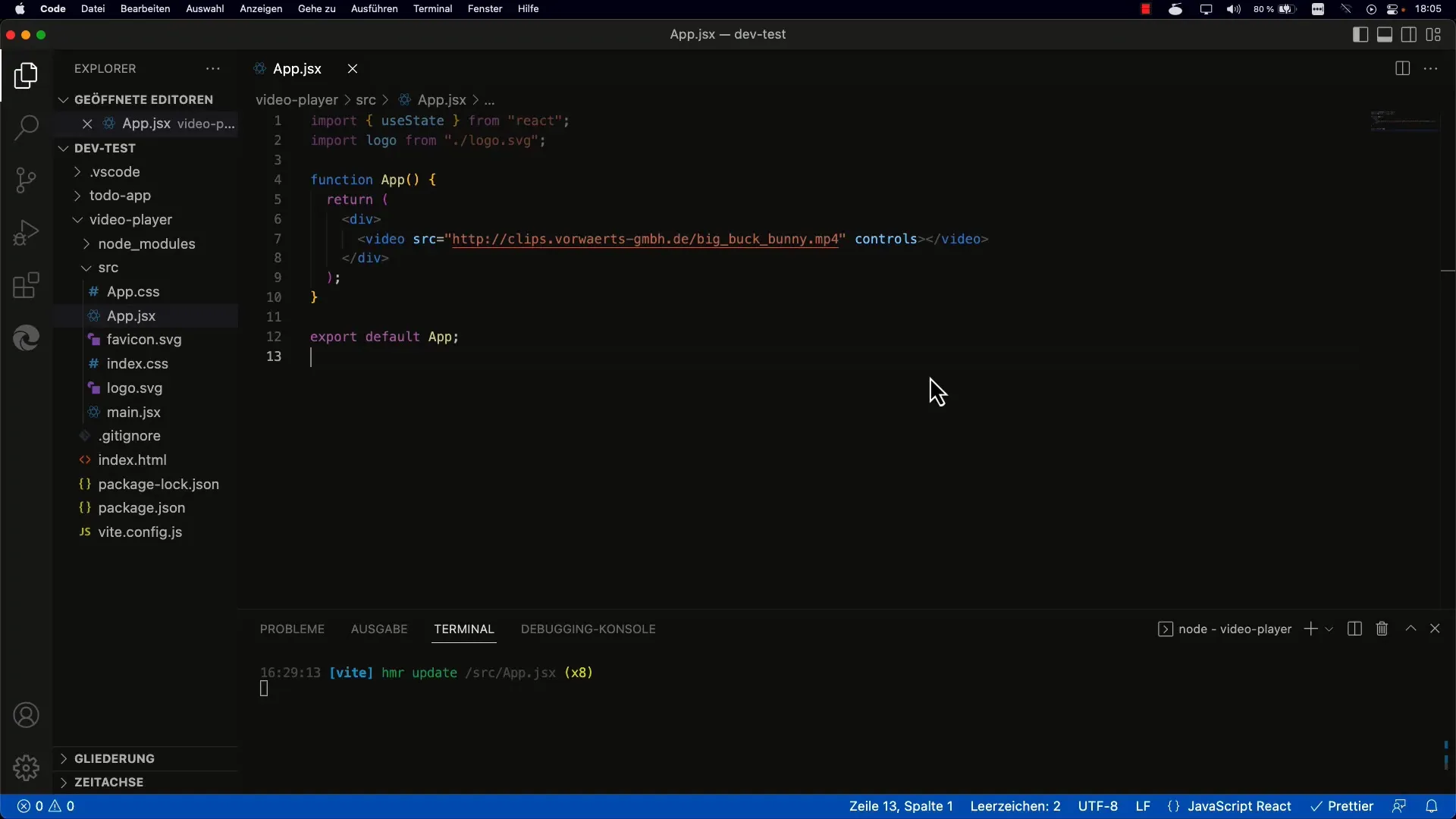
You can also enable the standard controls by adding the controls attribute. This allows you to play the video directly in the browser.
2. Accessing the Video Element with useRef
To access the video element with useRef, you need to create a reference in your component logic. Use const videoRef = useRef(null); and add the ref attribute to the
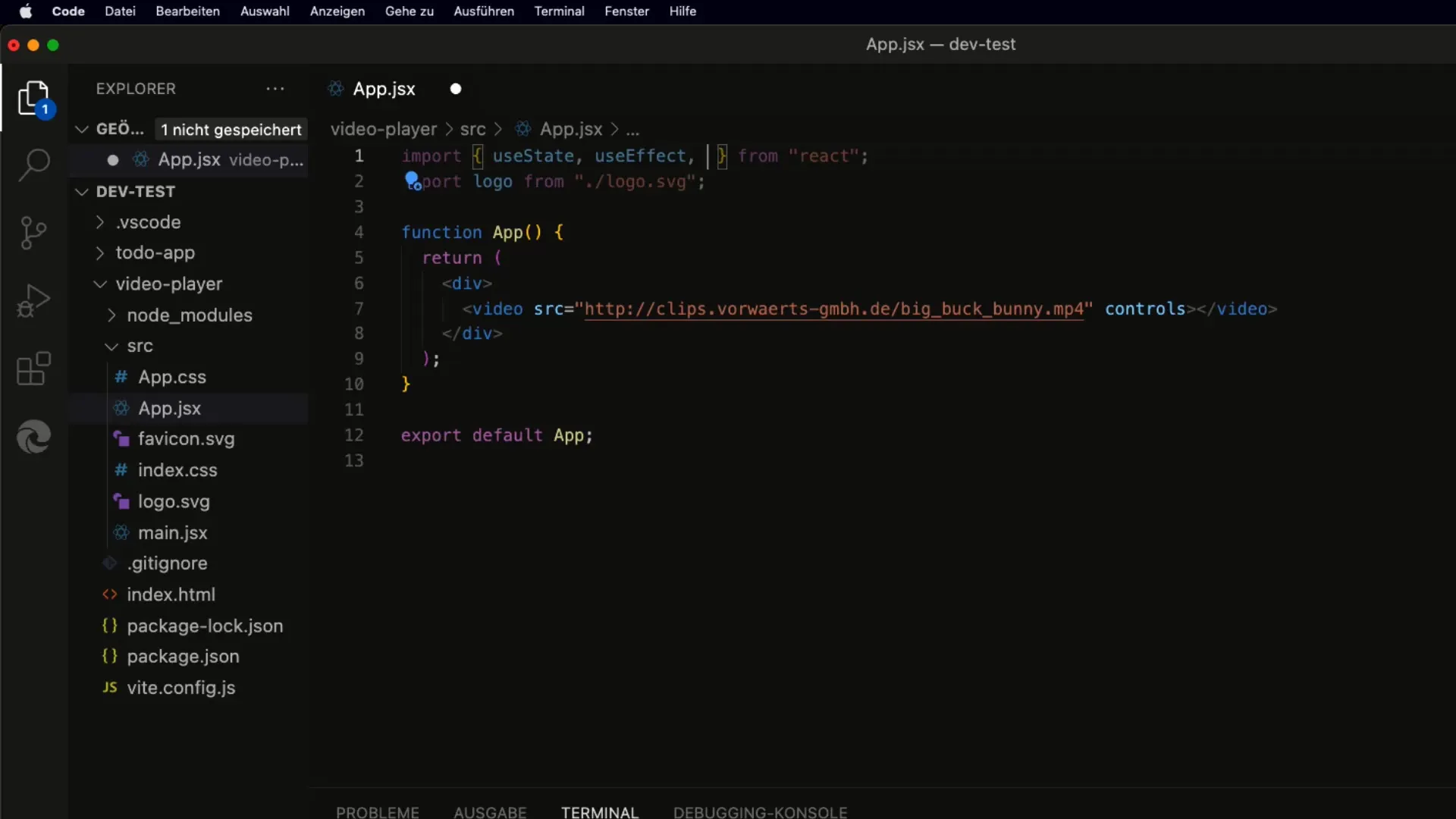
Now you have created a reference pointing to your video element.
3. Setting the Reference After the First Rendering
To actually use the reference, you need to ensure it is correctly set after the first rendering. For this, we use the useEffect Hook, which is called after the component is rendered. Add a console.log command to verify if the reference is correct.
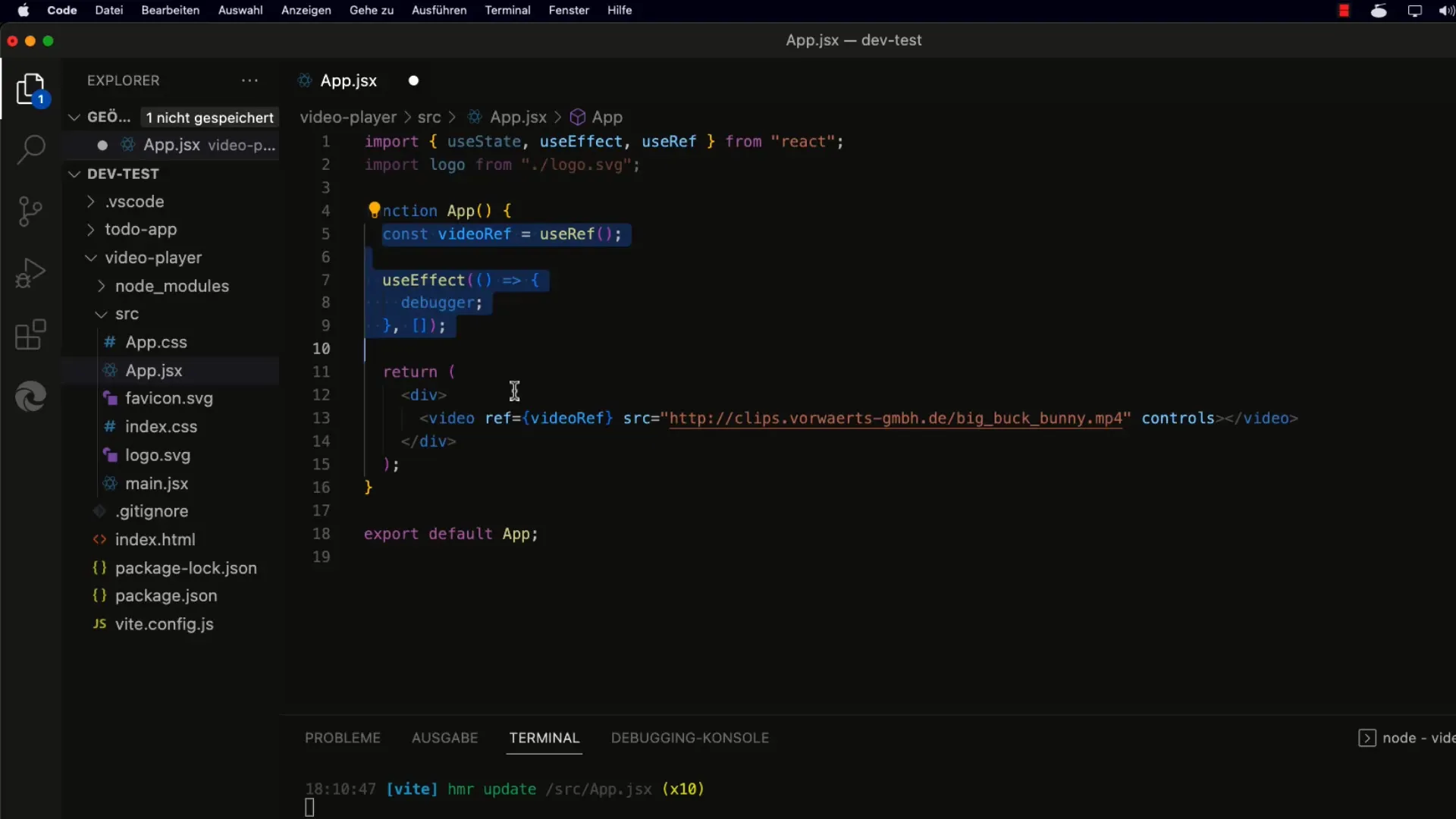
Now you can see if videoRef.current contains the video element.
4. Playing and Pausing the Video
To play the video, use the play() method. Note that this returns a Promise. It's also important to work with user interaction at some point to play the video. Add a button that allows the user to start the video.
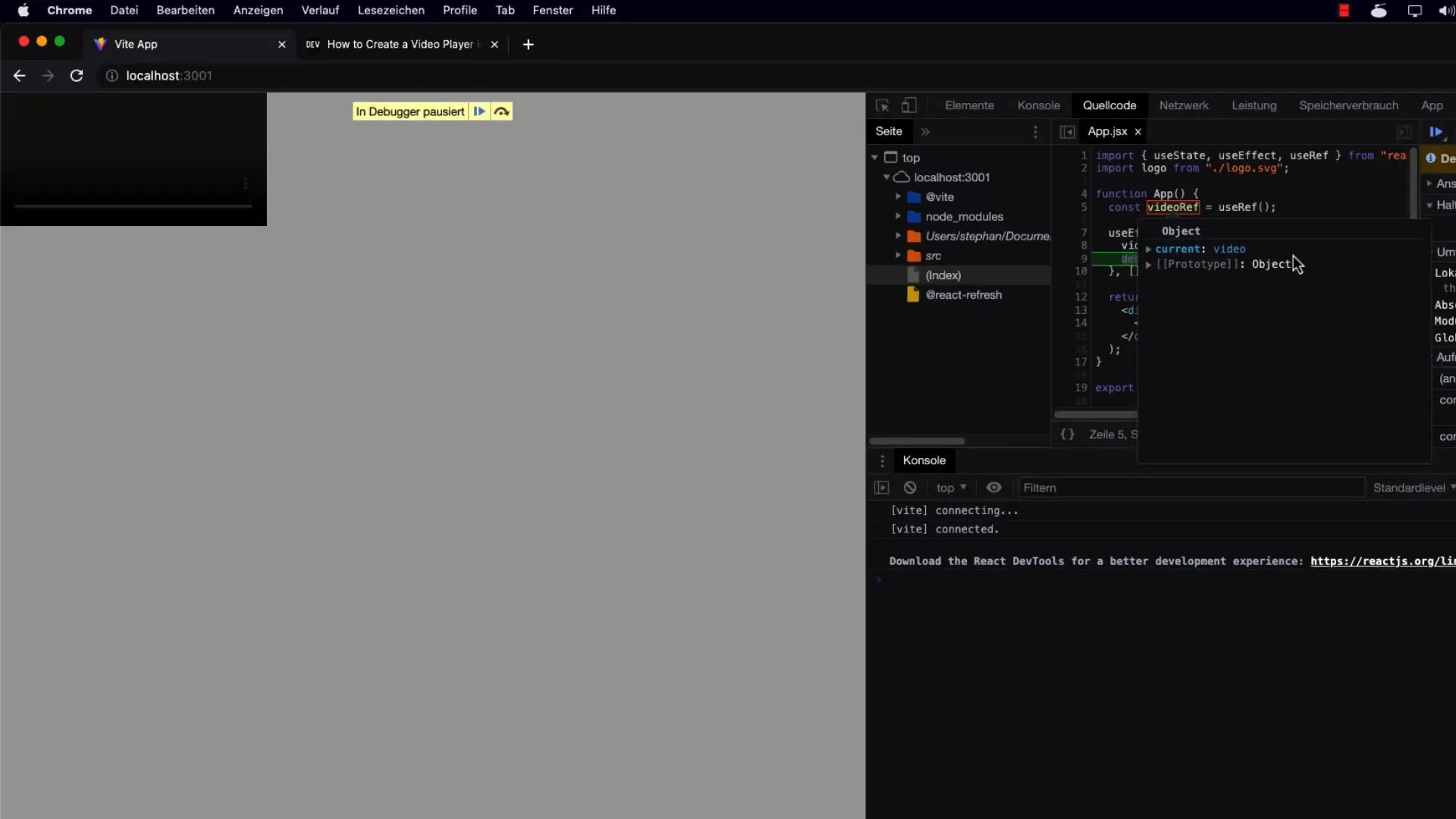
Make sure the user interacts with the page so the video can play correctly. If the video is not stopped, you may encounter an error message.
5. Muted Attribute for Autoplay
If you want the video to play automatically, the video element must be muted in the layout. Simply add the muted attribute to your
6. Summarizing the Steps and Features
Summarize what you have learned: you have inserted a video element into your app, created a reference with useRef, set the reference after rendering, and used the play() method. User interaction and the muted attribute for videos that should play automatically are essential.
Summary
Overall, you have learned how to control the video element in React using useRef and useEffect. The ability to set references to DOM elements is a powerful technique for creating custom controls for videos and enhancing your user experience.
Frequently Asked Questions
How do I use useRef for another DOM element?You can use useRef for any DOM element, just like you did for the video element.
How can I change the volume of the video?You can control the volume through the volume property of videoRef.current.
What should I do if the video is not playing?Ensure that user interaction has occurred and check if the video is set as muted.
Can I control multiple video elements with useRef?Yes, you can create multiple references by making multiple useRef calls and assigning each video element its own reference.