Come and dive into the world of React! You will learn how to react to changes in the src prop of your Video Player and effectively reset the player's state. This tutorial will show you how to correctly manage the state of your video player to ensure a smooth user experience.
Key Takeaways
- Use useEffect to react to prop changes.
- Reset multiple states when the src prop is changed.
- Ensure additional parameters like volume and position are initialized correctly.
Step-by-Step Guide
To implement the desired functionalities, we start by integrating and configuring the useEffect hook.
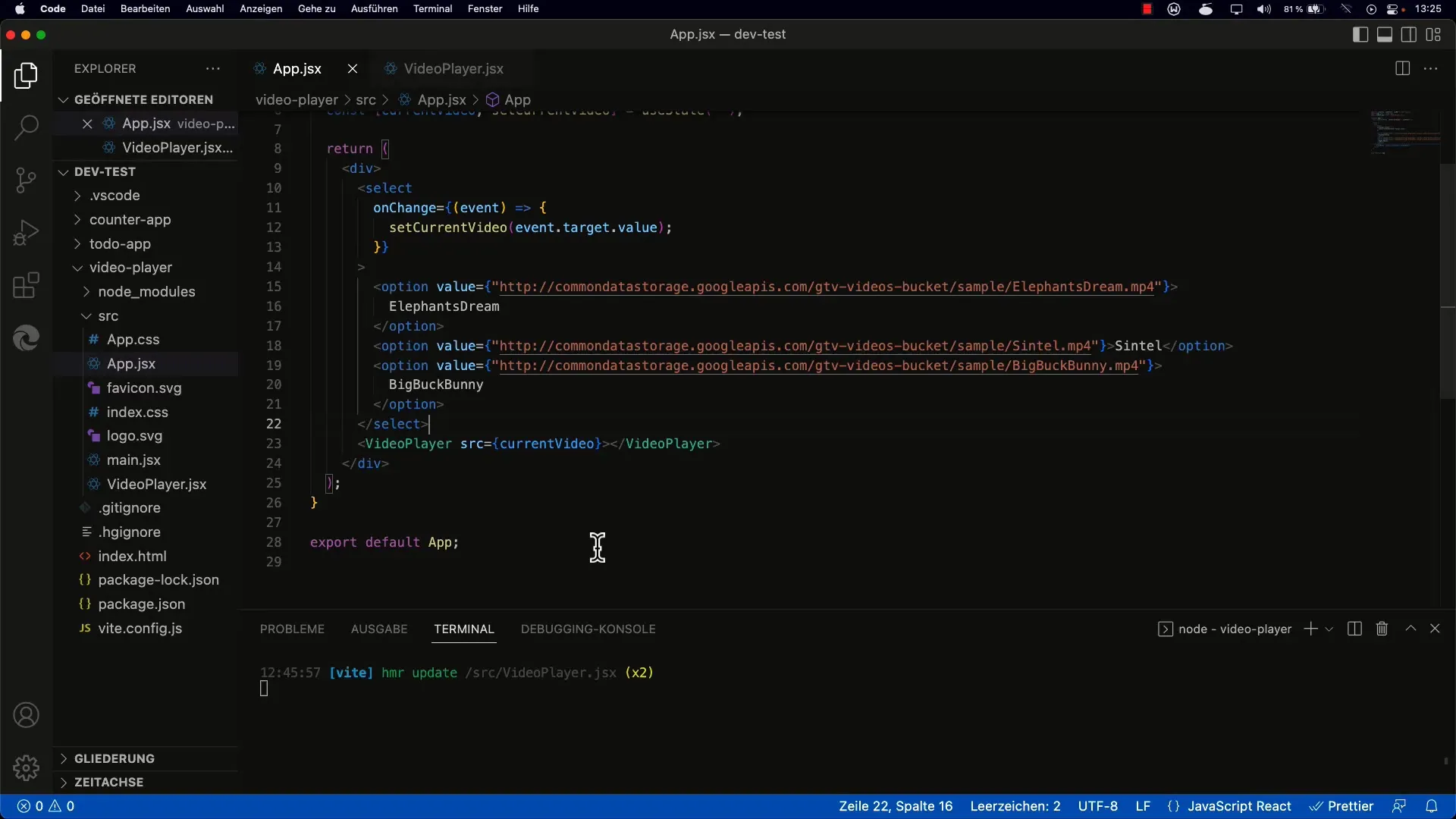
You should ensure that your video player is initially configured correctly. In the current implementation, it has been noticed that the player's state is not correctly updated when the video is changed.
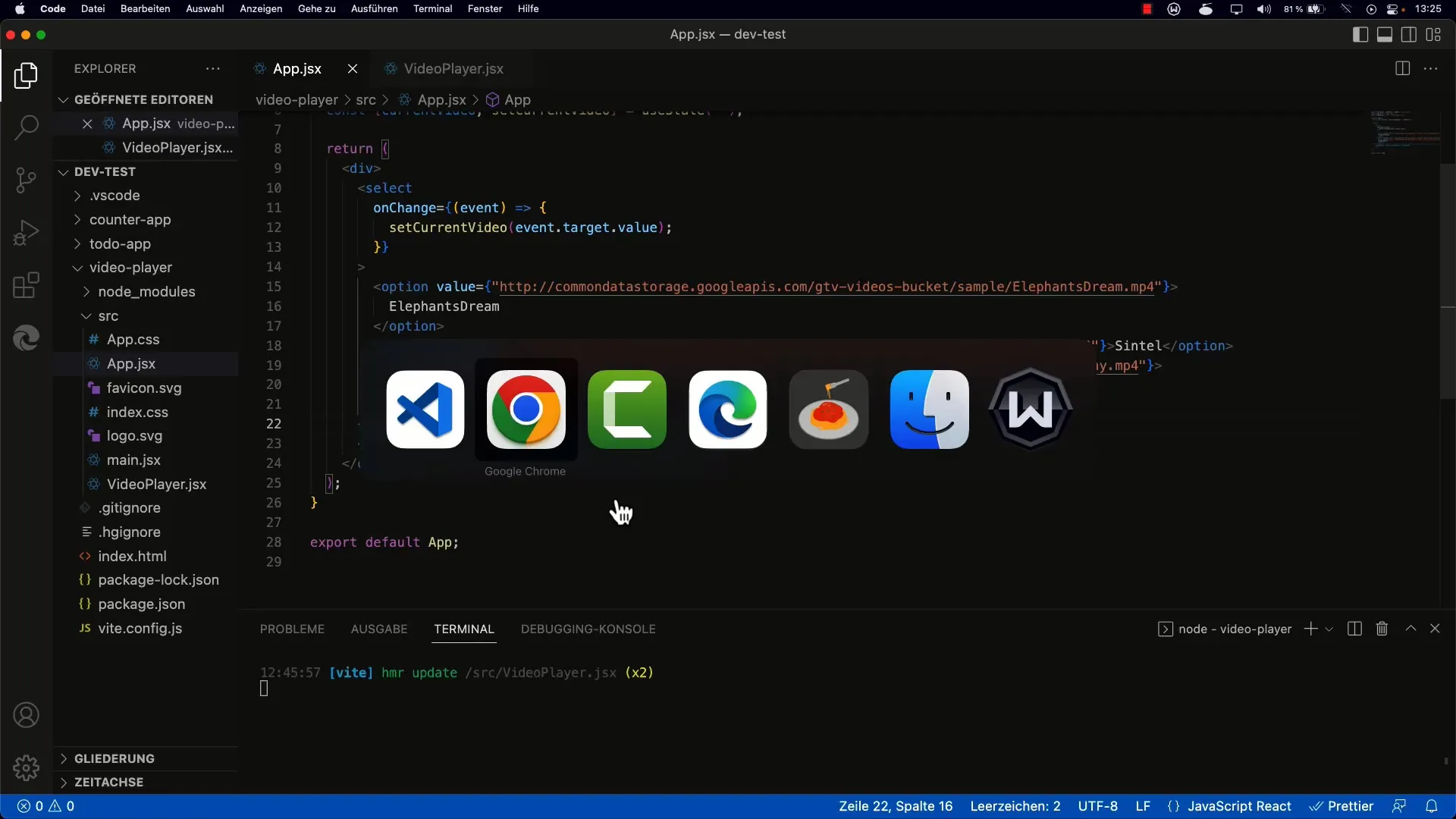
The main focus is on the src prop of the video element. When you change the video, the player's state, which contains information about the playback status, must be reset.
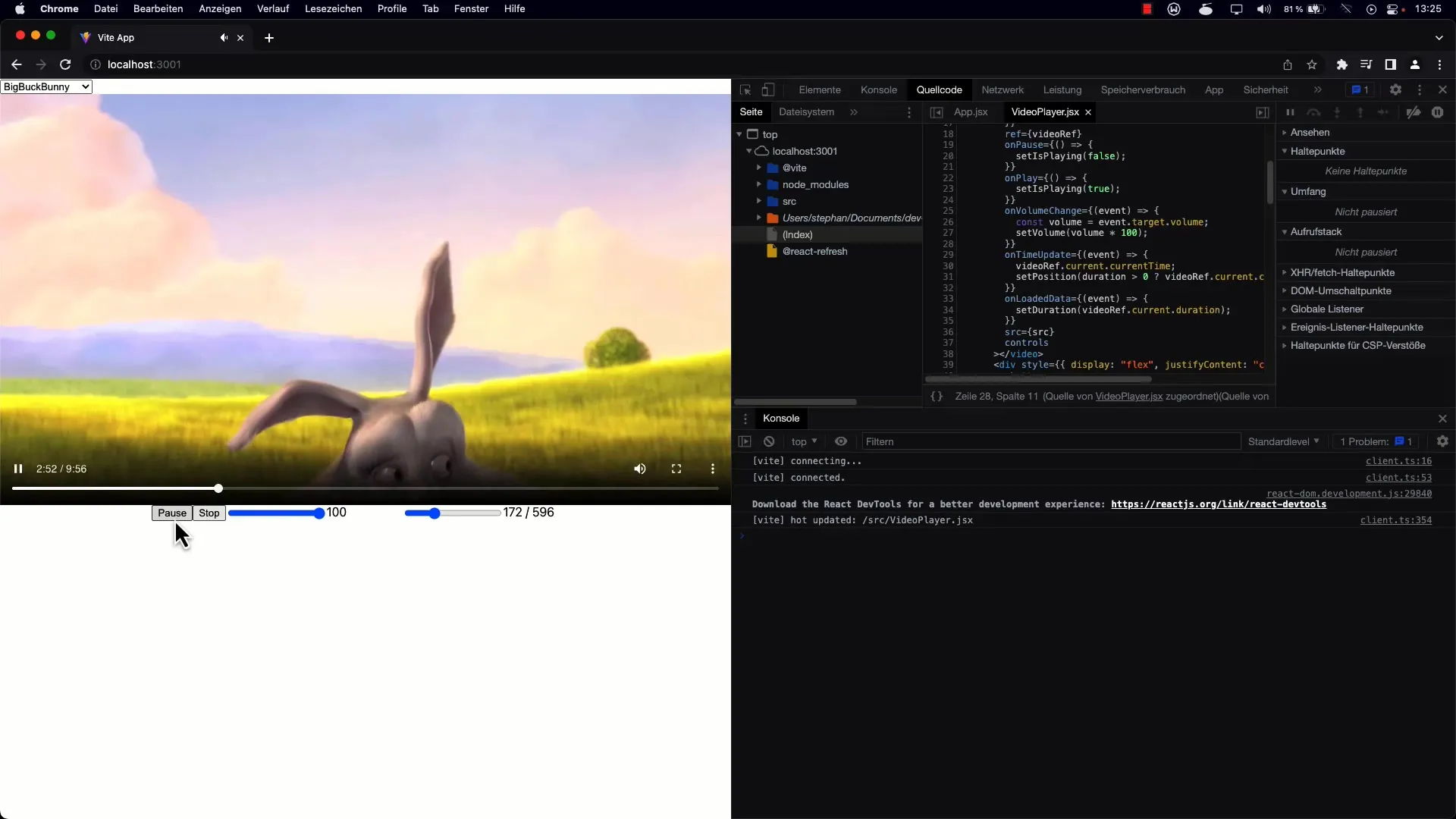
Open the component where you want to implement the logic and make sure the useEffect hook is imported. Here, you can leave an empty array as a dependency temporarily.
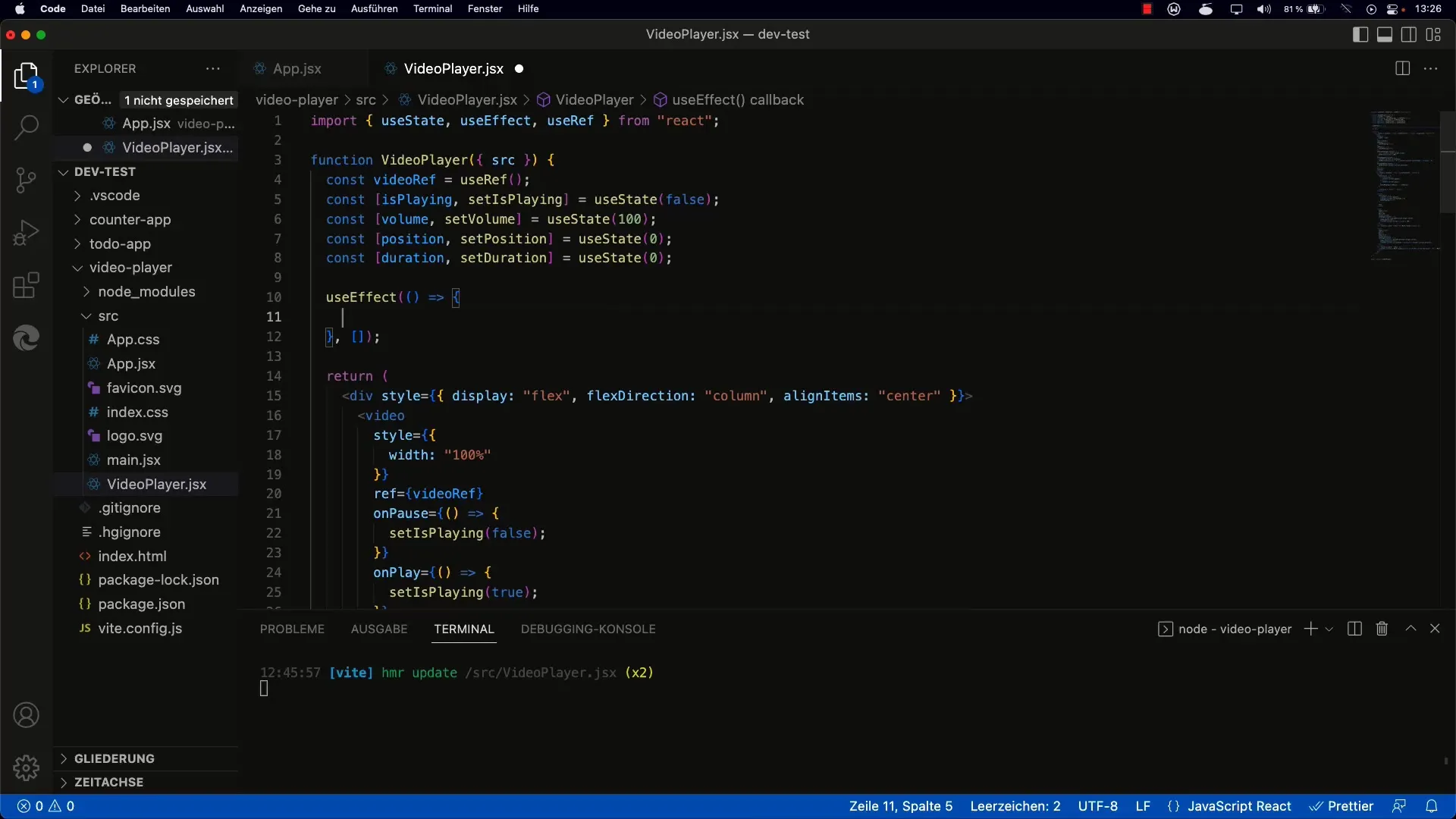
Now you will adjust the useEffect accordingly. You specify src as a dependent variable so that React can react to changes. This allows you to reset the state when the src prop changes.
For the reset process, you will define multiple states including isPlaying, duration, volume, and position. Set isPlaying to false when a new video is selected to ensure the player functions correctly upon restart.
Additionally, you need to reset the video's duration to zero and configure the volume at the maximum value of 100. Note that the volume property of the video element ranges from 0 to 1, so you set it to 1.
Also remember to reset the video's position. This ensures that when playing a new video, the progress is not carried over from the previous video.
After everything has been reset, reload your application to verify that the changes take effect.
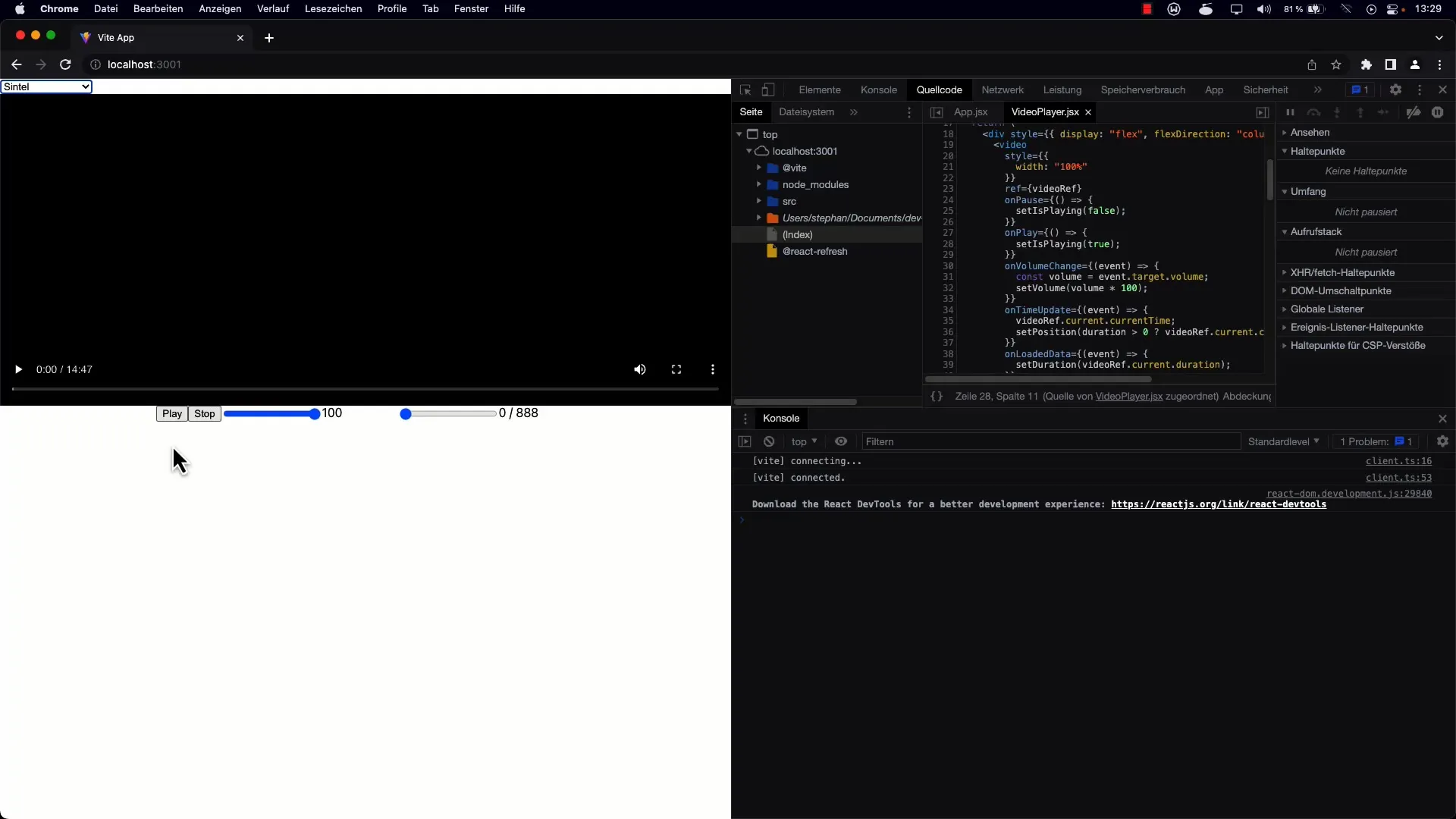
Select a video and play it. Then test changing the video to ensure that all resets function as expected.
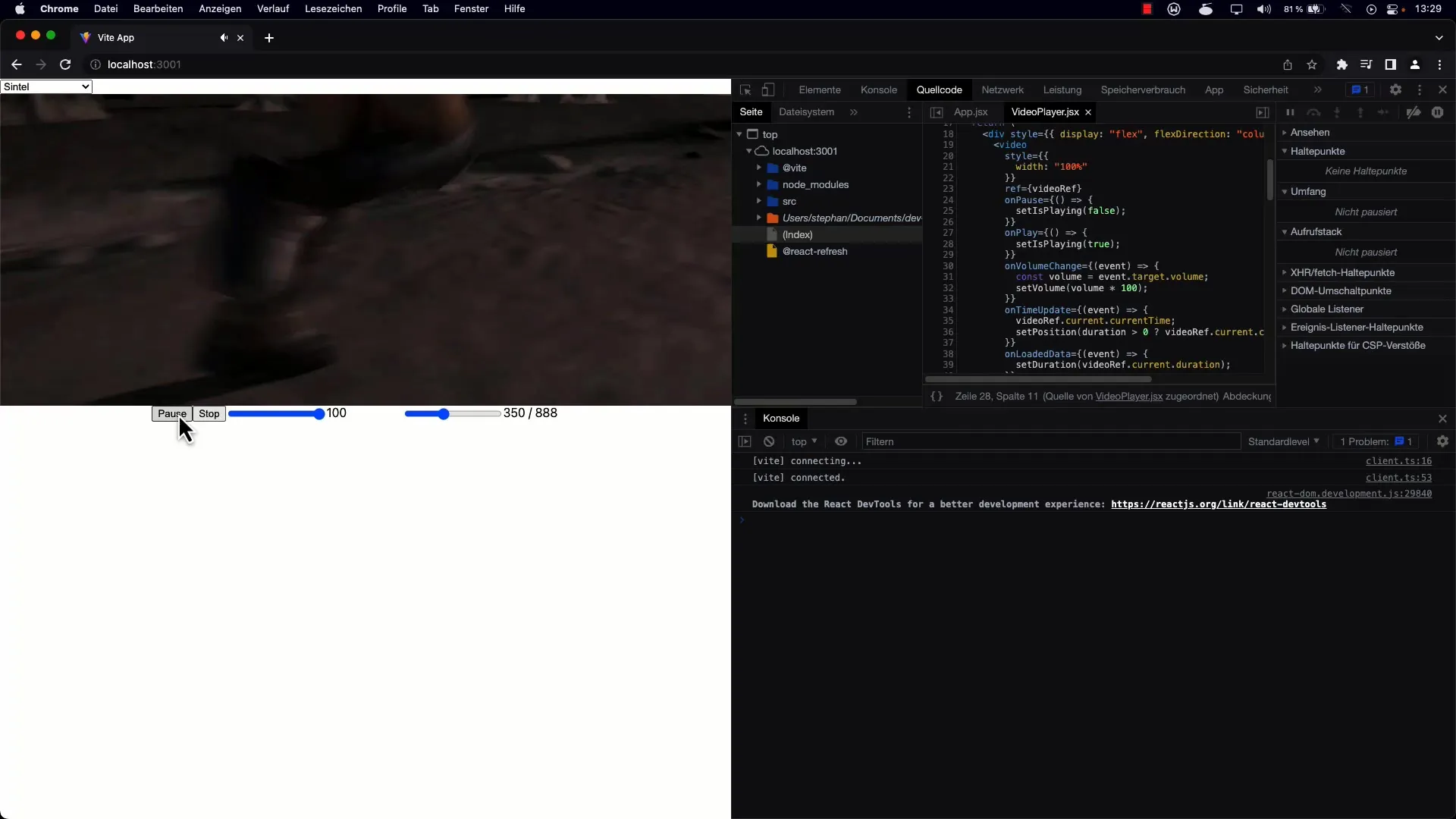
You will notice that after changing the video, the player's state is now correctly reset: the volume, playback position, and playback status are all correct. This ensures reliable usage.
If you notice that the volume is sometimes not reset to 100%, you need to readjust here. Make sure to also set the video element's volume to 100% after increasing the volume to ensure the user receives clear audio output.
Test the changes by changing the video again and checking the playback functions.
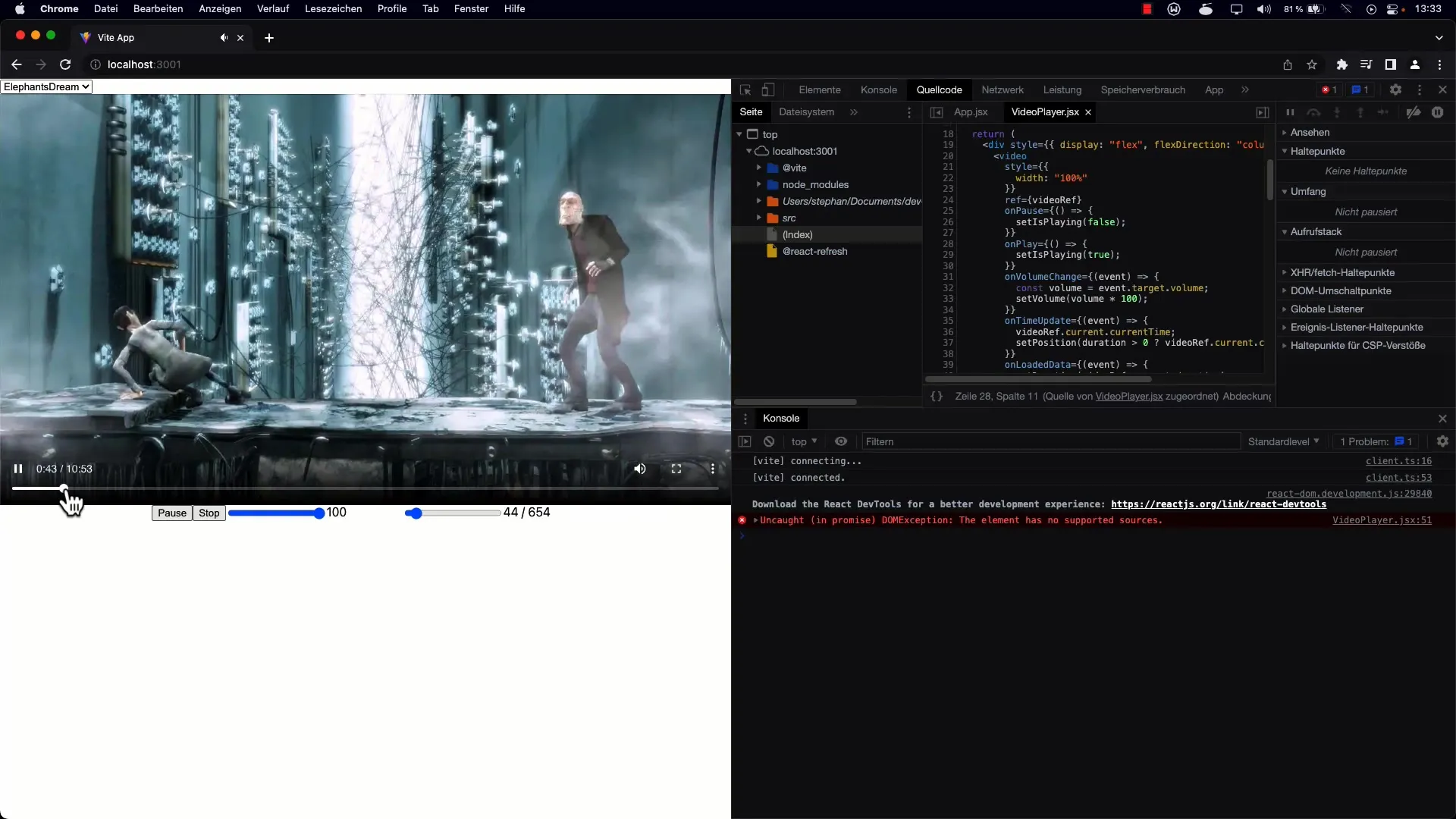
Furthermore, it is important to rectify any potential errors that may have occurred, such as incorrect setting of the src value. Therefore, regularly test the page to ensure that everything is configured as intended when the URL is initially loaded.
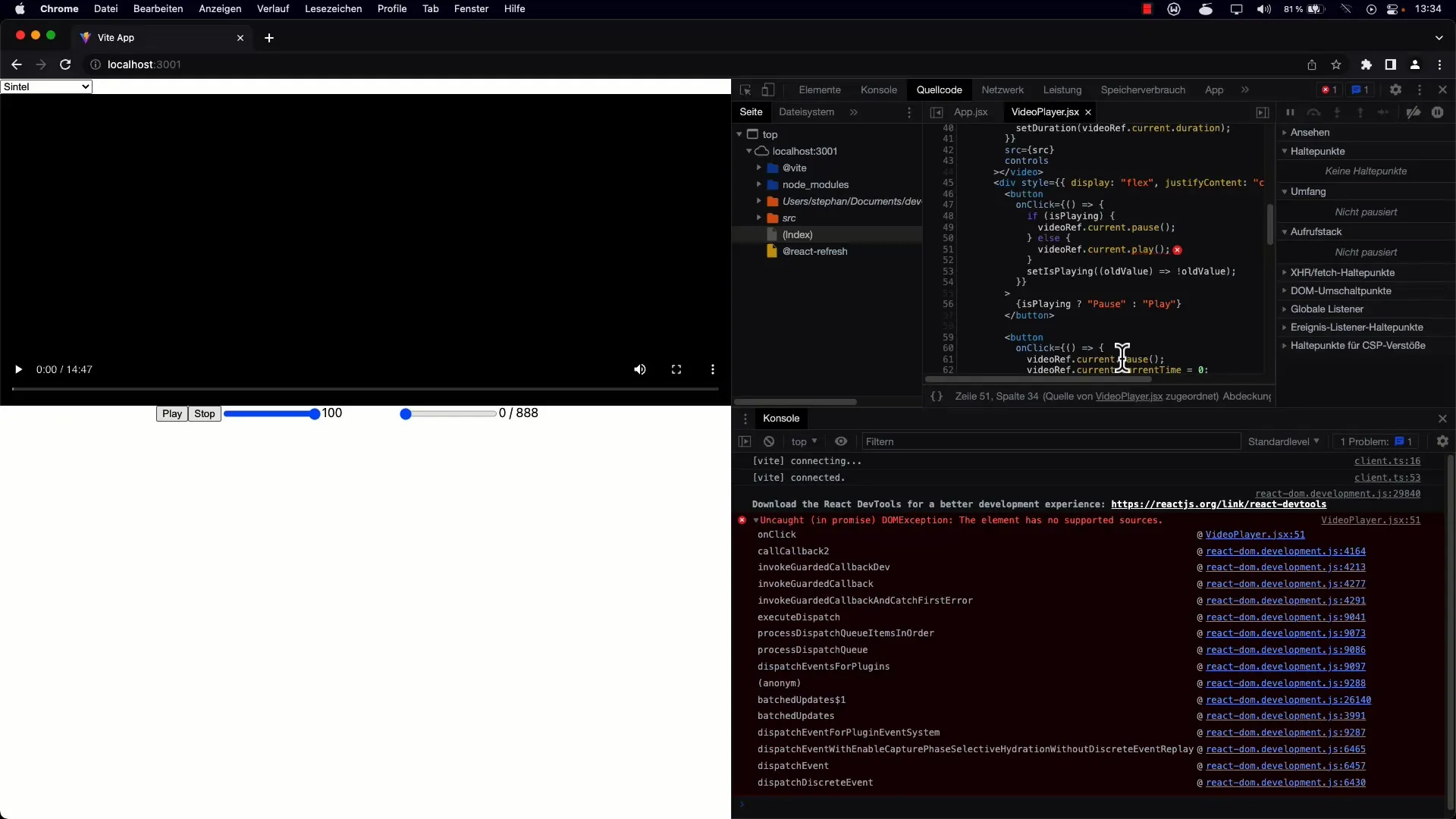
With these adjustments, you have ensured that your video player is correctly reset when the src is changed. This use of useEffect demonstrates effective handling of component effects in React.
Summary
In conclusion, you have learned how to manage and reset the state of your video player using the useEffect hook when the src prop changes. This important technique helps you create a seamless and user-friendly experience.
Frequently Asked Questions
How can I ensure that the volume value is reset correctly?You need to set the volume of the video element to 1 to ensure that the volume is at its maximum, as the value ranges from 0 to 1.
What does the useEffect hook do in this situation?The useEffect hook allows you to respond to changes in the src prop, so that all dependent component values can be reset.
What happens if the src prop is empty?If the src prop is empty, the video playback will not start, and you may encounter an error. Make sure to set a default value.