Oftentimes we find in software development that even small changes to the user interface can have a big impact on usability. In this tutorial, we focus on how to disable the navigation buttons - Next and Previous - in a React application when they are not usable. This simple but important detail enhances interaction and leads to a smoother user experience.
Key Takeaways
- The buttons for "Next" and "Previous" can be effectively disabled to indicate that no actions are possible.
- A new functional design is necessary to review the current navigation options.
- By implementing state checks, we can improve the visibility and effectiveness of controls.
Step-by-Step Guide
Step 1: Analyze the Buttons
First, let's look at the structure of the buttons. In this application, there are two specific buttons: one for "Skip Next" and one for "Skip Previous". The first step is to check whether these buttons are currently enabled or disabled on the user interface.
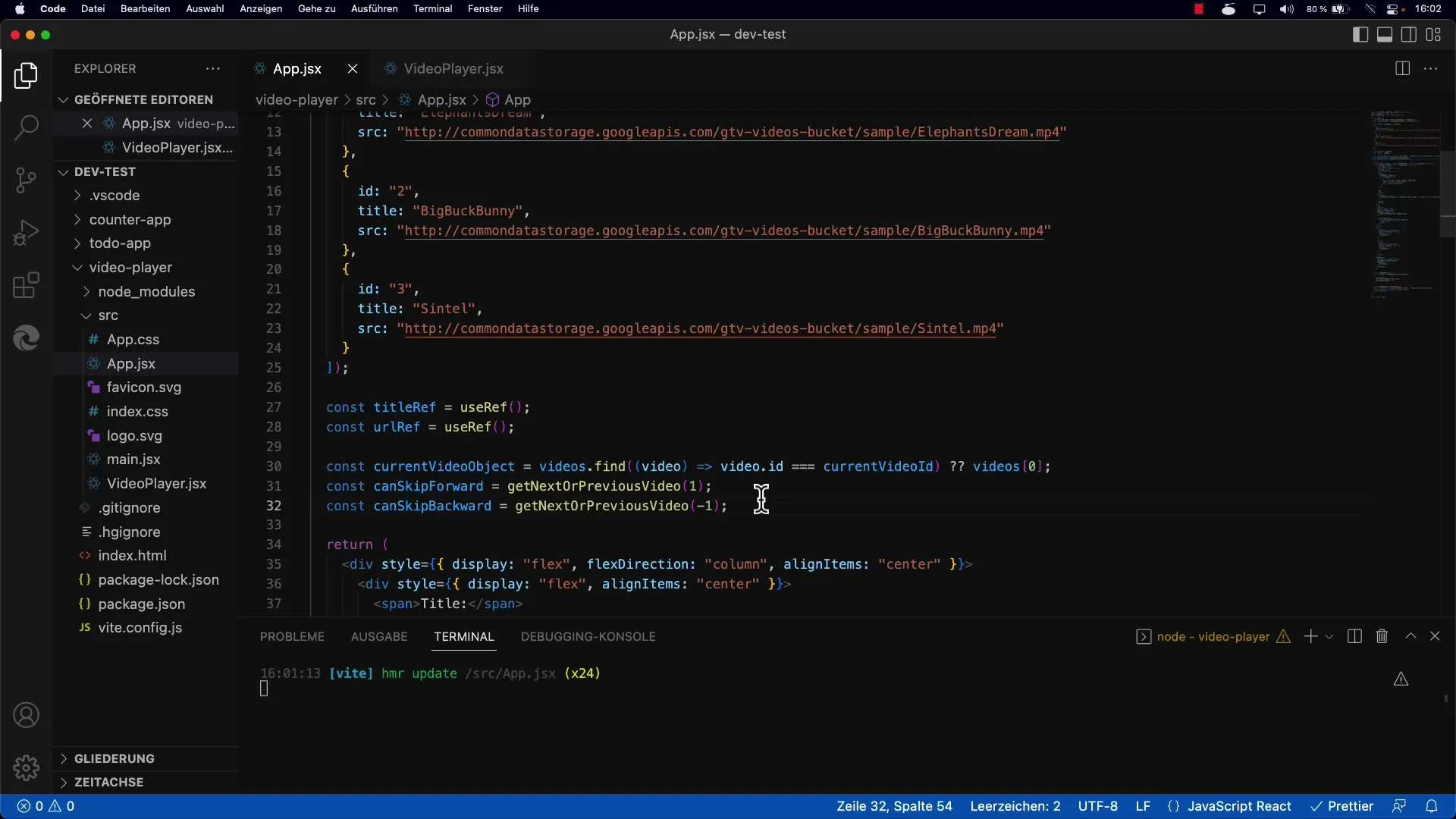
Step 2: State Check for the Buttons
Now, you will implement the logic that determines when the buttons should be disabled. This means you need to define certain variables in your state that indicate whether skipping backward or skipping forward is possible. It's useful to use the variables skipBackward and canSkipForward.
Step 3: Implementation of Logic in the Code
To dynamically disable the buttons, you check the aforementioned variables in your render area. For example, if the variable skipBackward has a value of false, the "Previous" button should be disabled.
Step 4: Introduce a New Function
To make the logic clearer and more modular, you extract the logic into a separate function. This new function, getNextPreviousVideo, takes a parameter indicating whether to retrieve the next or previous video.
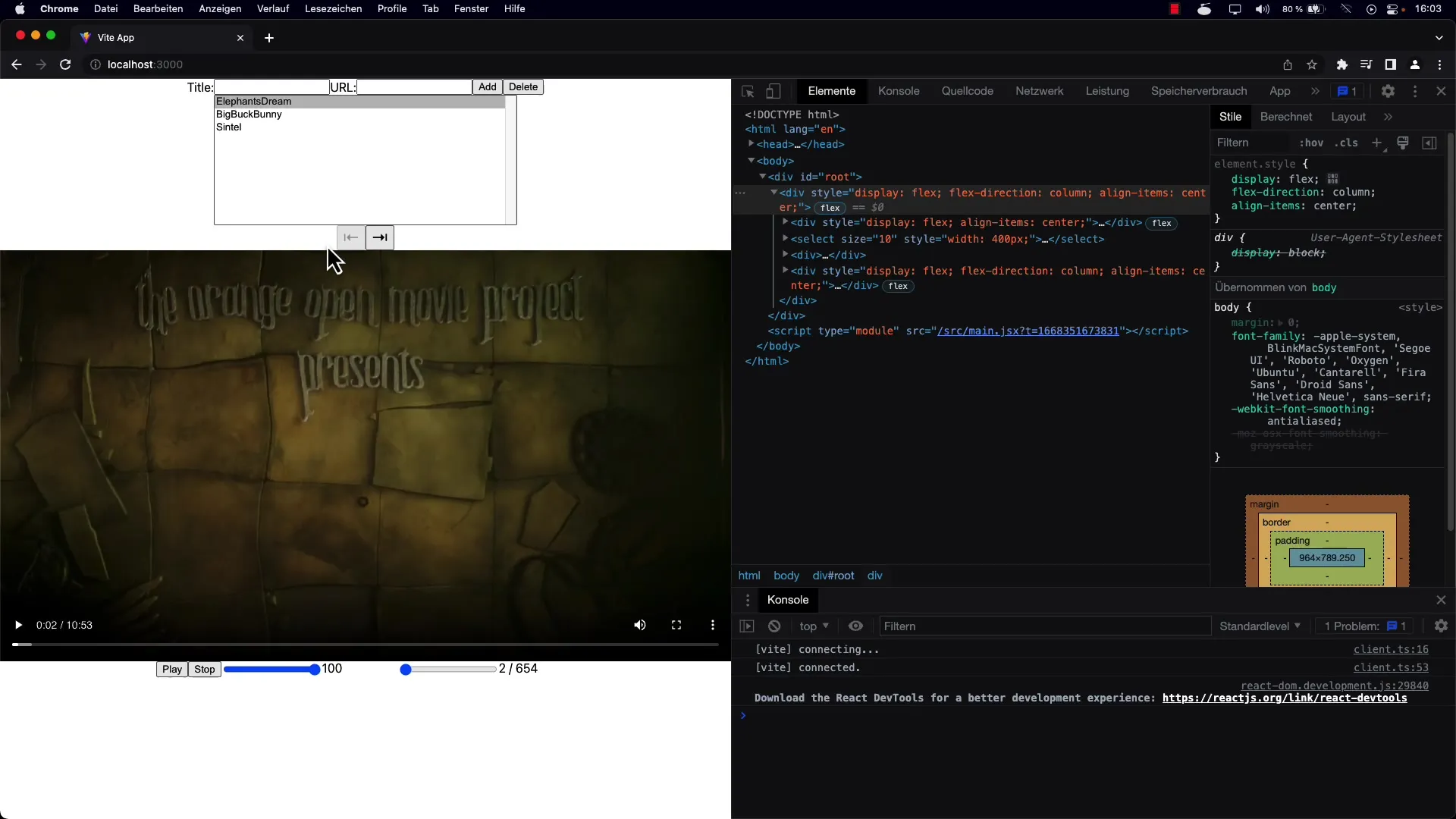
Step 5: Use the New Function
This redesigned function returns the corresponding video and sets the video ID accordingly. You can decide whether to increase or decrease the current state by one step to render the next or previous video.
Step 6: Consolidate Conditions
Now add the condition for the button state to also consider the return of the new function. Ensure that if the returned video is undefined, the buttons are disabled accordingly.
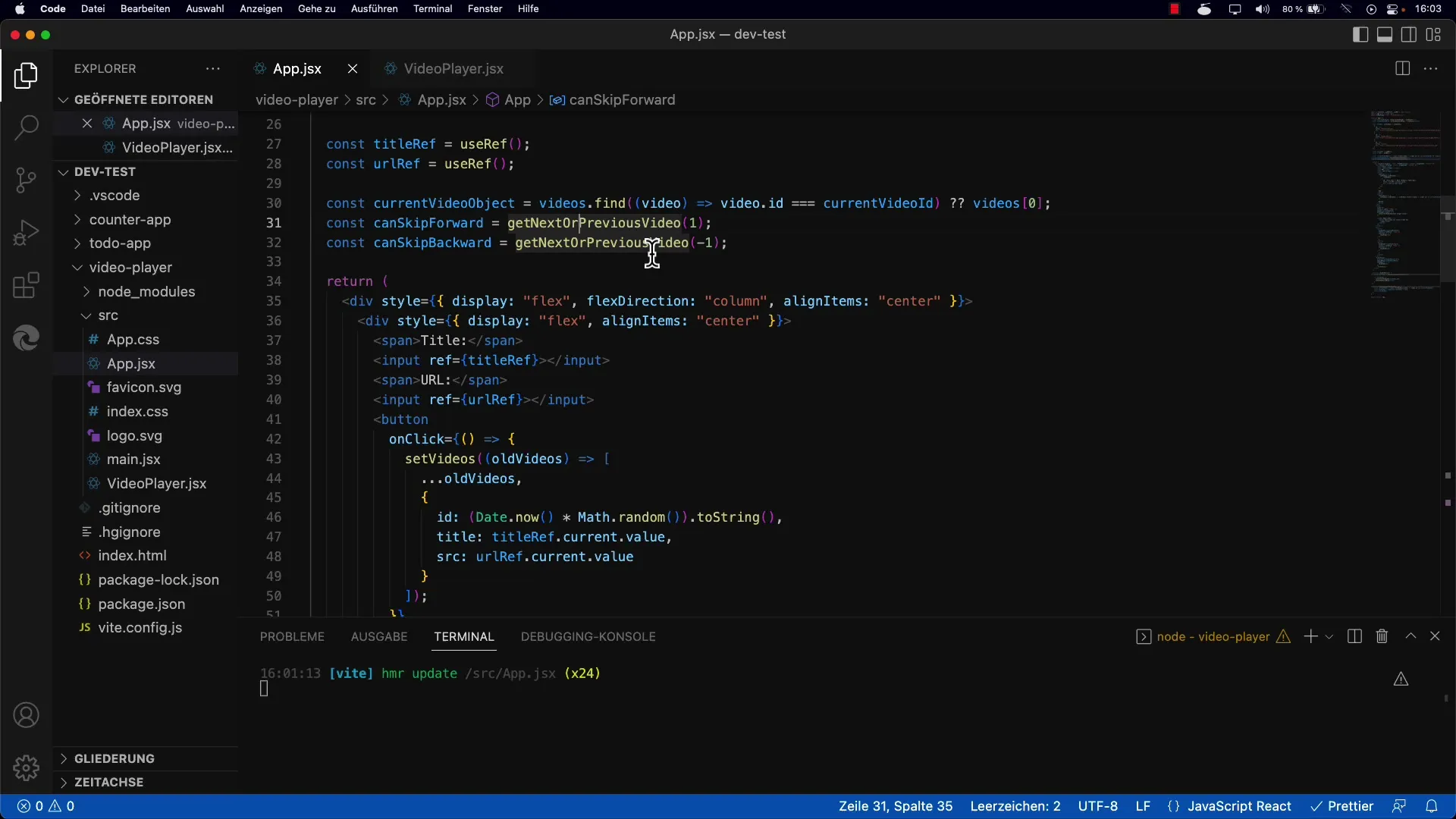
Step 7: Test the Implementation
After implementing, start the application and test the functionality of the buttons. Click through the videos and check if the buttons are correctly disabled when you are at the beginning or end of the list.
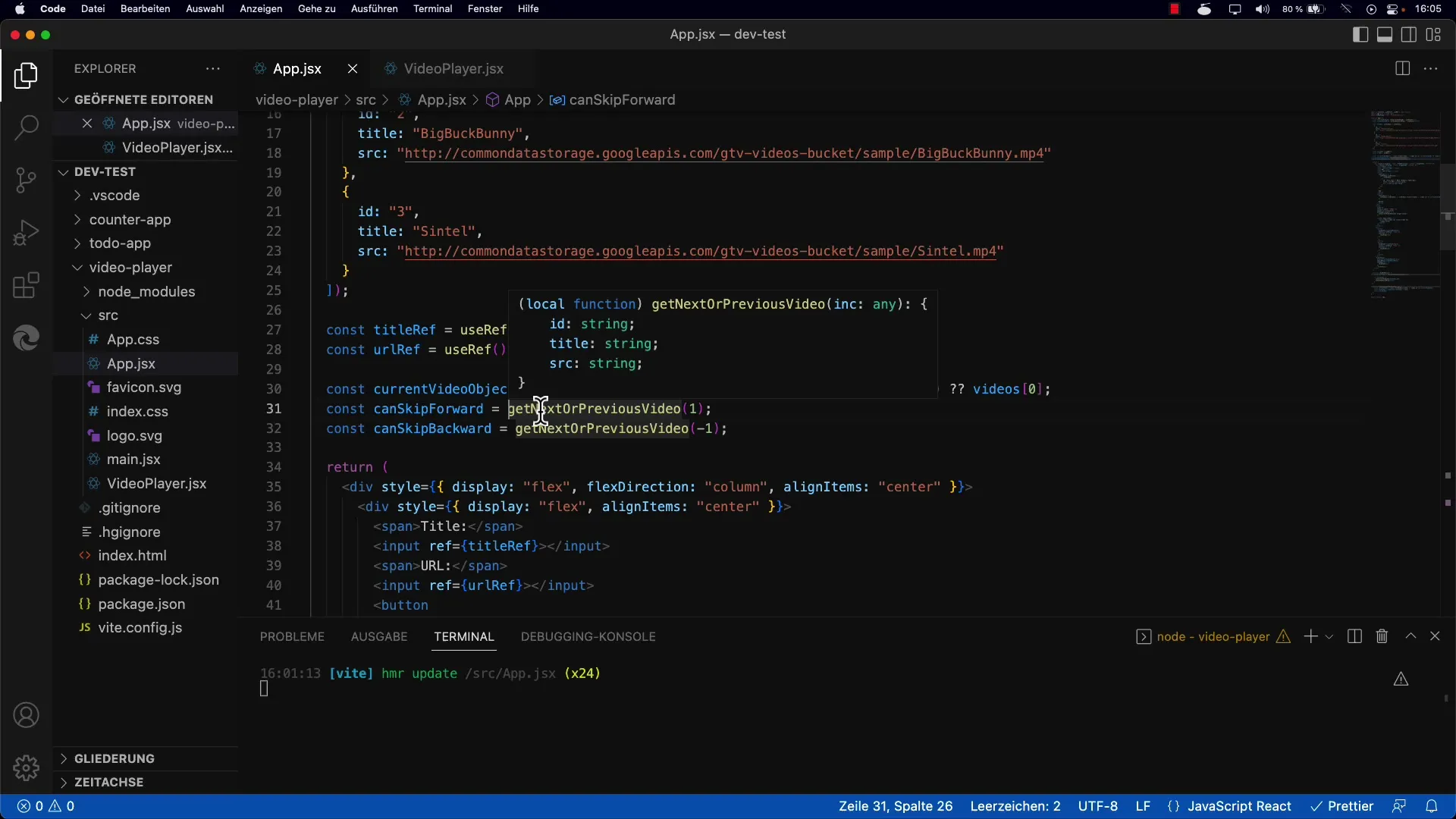
Step 8: Focus on User Experience
To ensure an effective user experience, these small details are crucial. By disabling non-functioning buttons, the application clearly signals which actions are available and which are not. This is crucial for usability, especially for new users.
Summary
This tutorial has shown you how to improve navigation in a React application by disabling the Next and Previous buttons for unauthorized actions. Implementing this functionality significantly enhances usability and ensures that users intuitively understand their available options.
Frequently Asked Questions
What does it mean when a button is disabled?A disabled button indicates that the corresponding action cannot currently be performed.
How can I implement the logic for disabled buttons in React?You can use state checks in your render method and link them to the variables of your navigation.
Where can I find more information about React buttons?The official React documentation provides extensive information on components and their state.