The use of custom hooks in React has established itself as a brilliant approach to abstract recurring logic and improve the readability and maintainability of your components. In this tutorial, you will learn how to create your own hooks through the example of "useJsonFetch", specifically designed for fetching JSON data from a server. Let's dive right in.
Key Takeaways
- Custom hooks are simple functions that use React hooks.
- They allow the reuse of logic across multiple components.
- A custom hook can handle multiple states like loading, data, and error.
- The structure and implementation of a custom hook is straightforward and manageable.
Step-by-Step Guide to Creating the useJsonFetch Hook
Basic Considerations
Before writing your own hook, it is important to define the functionality you need. In this case, you want to create a hook that fetches JSON data from a server. We will name our hook "useJsonFetch". To understand how to implement this hook, first look at the usage of useJsonFetch in a component.
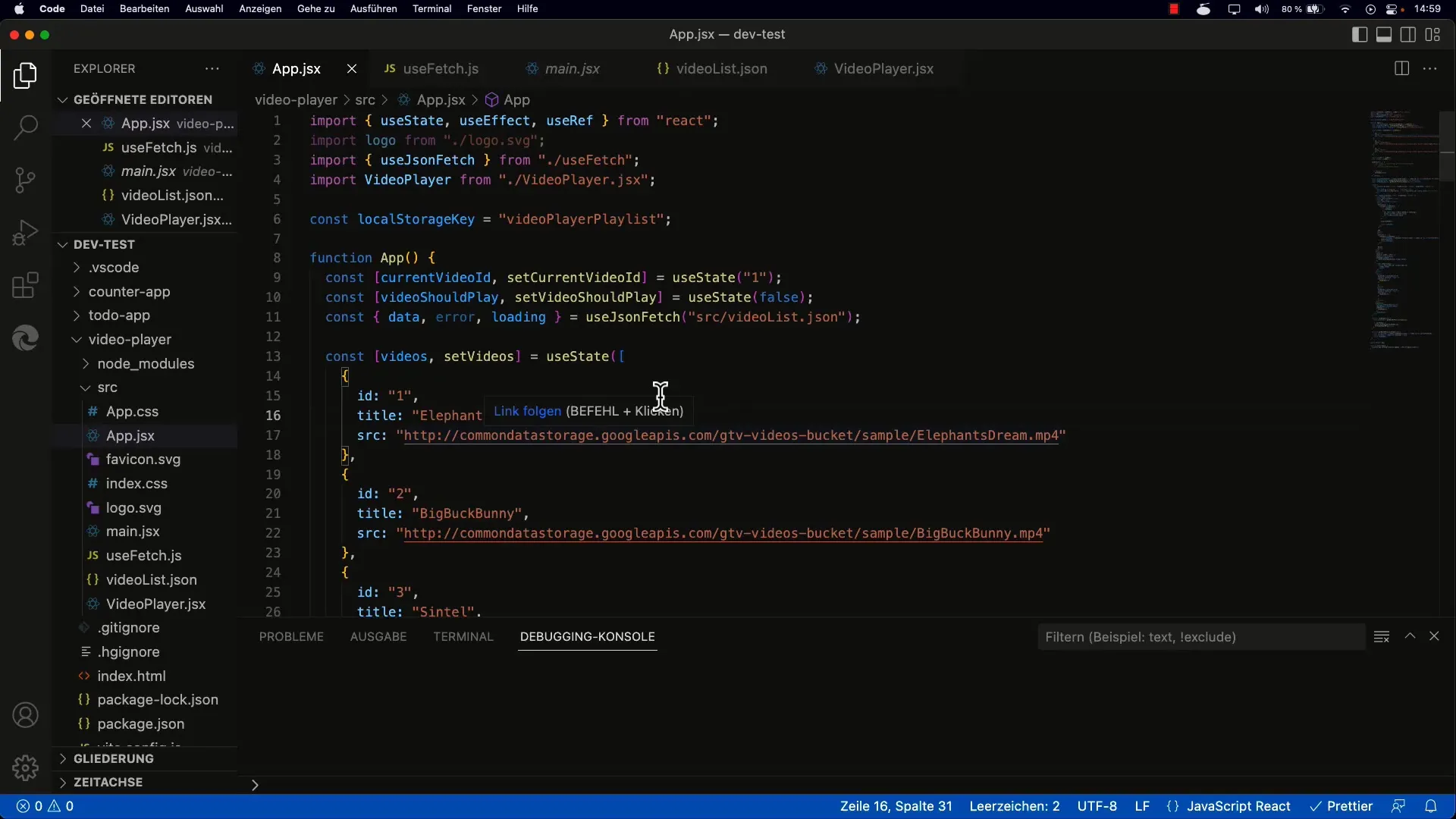
You call the hook and pass the URL from which you want to fetch the JSON data. The hook will be responsible for fetching the data and handling loading and error states.
Implementation of useJsonFetch
To implement the custom hook, create a new file that starts with "use" to comply with React conventions. So, the file name would be useJsonFetch.js.
In this file, create a function that takes the URL as a parameter. In the function, define the states for data, error, and loading.
Here, data is initially undefined, error is also undefined, and loading starts as true to indicate the loading state. This is due to how JavaScript handles asynchronous operations.
Using useEffect
Within the hook, you use useEffect to start the fetch process when the component is mounted. Make sure to update the loading state accordingly.
Using await, you first call the URL with fetch. Since fetch returns a Promise, you can await the response with await. After fetching the response, you convert the data to a JavaScript object using.json().
If an error occurs, you catch it with try-catch and set the error state. Regardless of whether the request was successful or an error occurred, make sure to set loading to false once the response arrives.
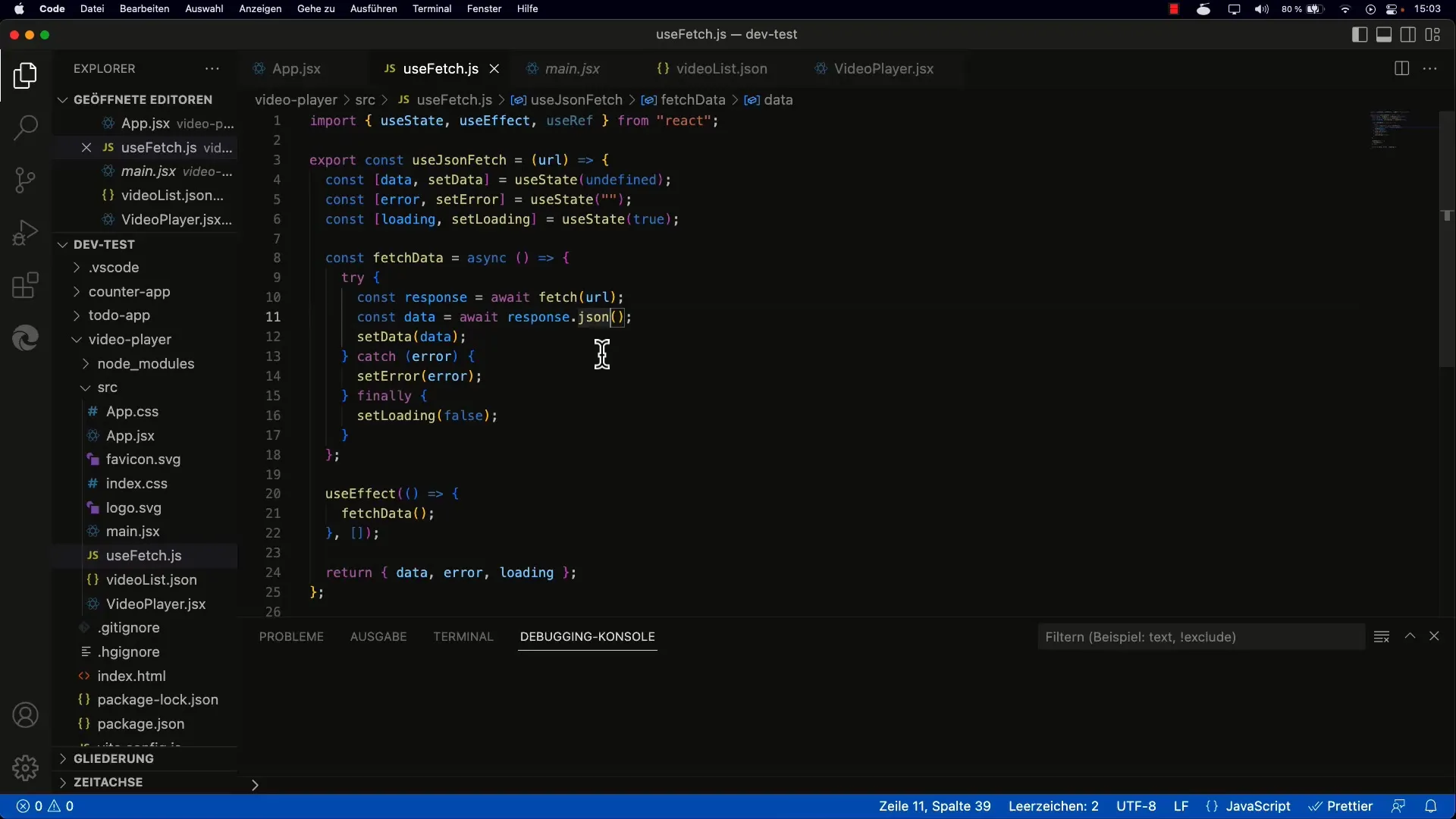
Return from the Hook
Finally, return the states data, error, and loading as an object so that the calling component has access to these values.
Using the Hook in a Component
Now that you have created your hook, you can use it in your component. Import useJsonFetch into your component and use destructuring to get the values.
You can then implement the loading indicator by checking loading and display the data you fetched when it is available.
By using useJsonFetch, you can maintain logical separation between data fetching and UI logic, significantly improving the maintenance of your components.
Conclusion on Creating Custom Hooks
Creating custom hooks is a simple yet powerful process that helps you organize your code. You can encapsulate reusable logic and implement it in any component needing the same functionality. By using custom hooks, you can also separate network calls and responses to loading or error states from displaying the data.
Summary
By using your own hook like useJsonFetch, you learn how to manage asynchronous data fetching in React. This significantly improves the reusability and structure of your code. You have seen how to build a simple structure to fetch JSON data and effectively manage the different states.
Frequently Asked Questions
What is a Custom Hook?A Custom Hook is a JavaScript function that uses React Hooks to encapsulate logic that can be reused in multiple components.
How do I implement the useJsonFetch Hook?Create a function in a new file that takes the URL as a parameter and uses React Hooks like useState and useEffect.
Why should I use Custom Hooks?Using Custom Hooks allows you to simplify recurring logic and organize your code. It improves the maintainability of your components.
Can I have multiple Hooks in one file?Yes, you can define and export multiple Hooks in one file as long as they fit a specific context or functionality.
How do I handle errors in a Custom Hook?You can catch errors with a try-catch block and update the Error-State accordingly.