Dealing with State in React can sometimes be a challenge, especially when it comes to understanding mutations and handling references correctly. When using the setter of useState, you often need to create new arrays or objects to ensure that React recognizes your changes. This can quickly become cluttered and require a lot of code. An alternative is useImmer, which allows you to modify the state in a much more intuitive way. In this guide, we will walk you through the process step by step of how you can use useImmer instead of useState.
Key takeaways
- useImmer is a replacement for useState that simplifies handling mutable state in React.
- With useImmer, you can make changes directly to arrays and objects without having to manually create new references.
- Mutations are automatically converted to new, immutable states, greatly simplifying handling.
Step-by-step guide
Installation of useImmer
First, you need to make sure that useImmer is installed. This is done via the terminal command:
npm install immer
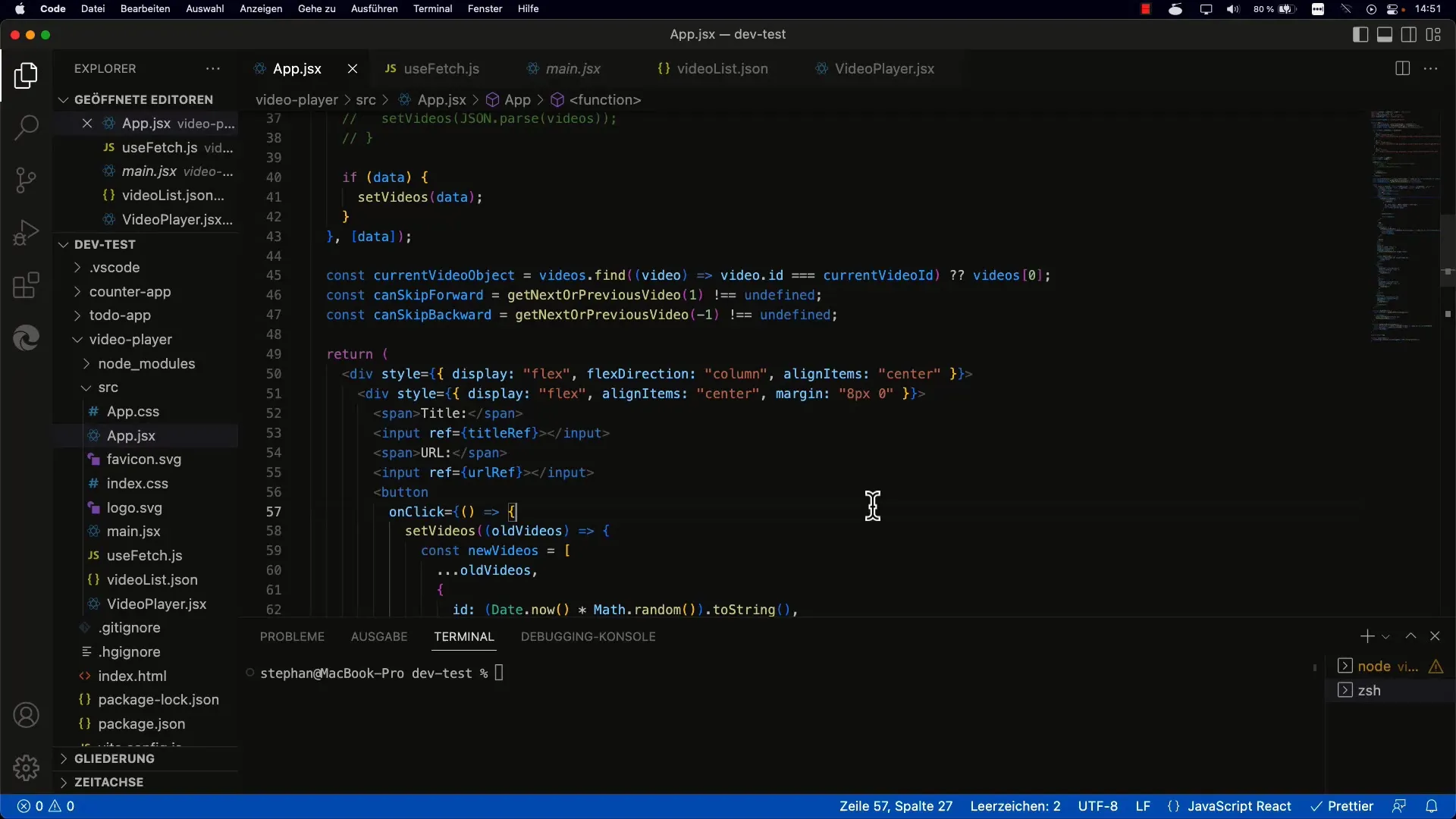
Once the installation is complete, you should check if the package appears correctly in your package.json file. You should always see it in the list of dependencies.
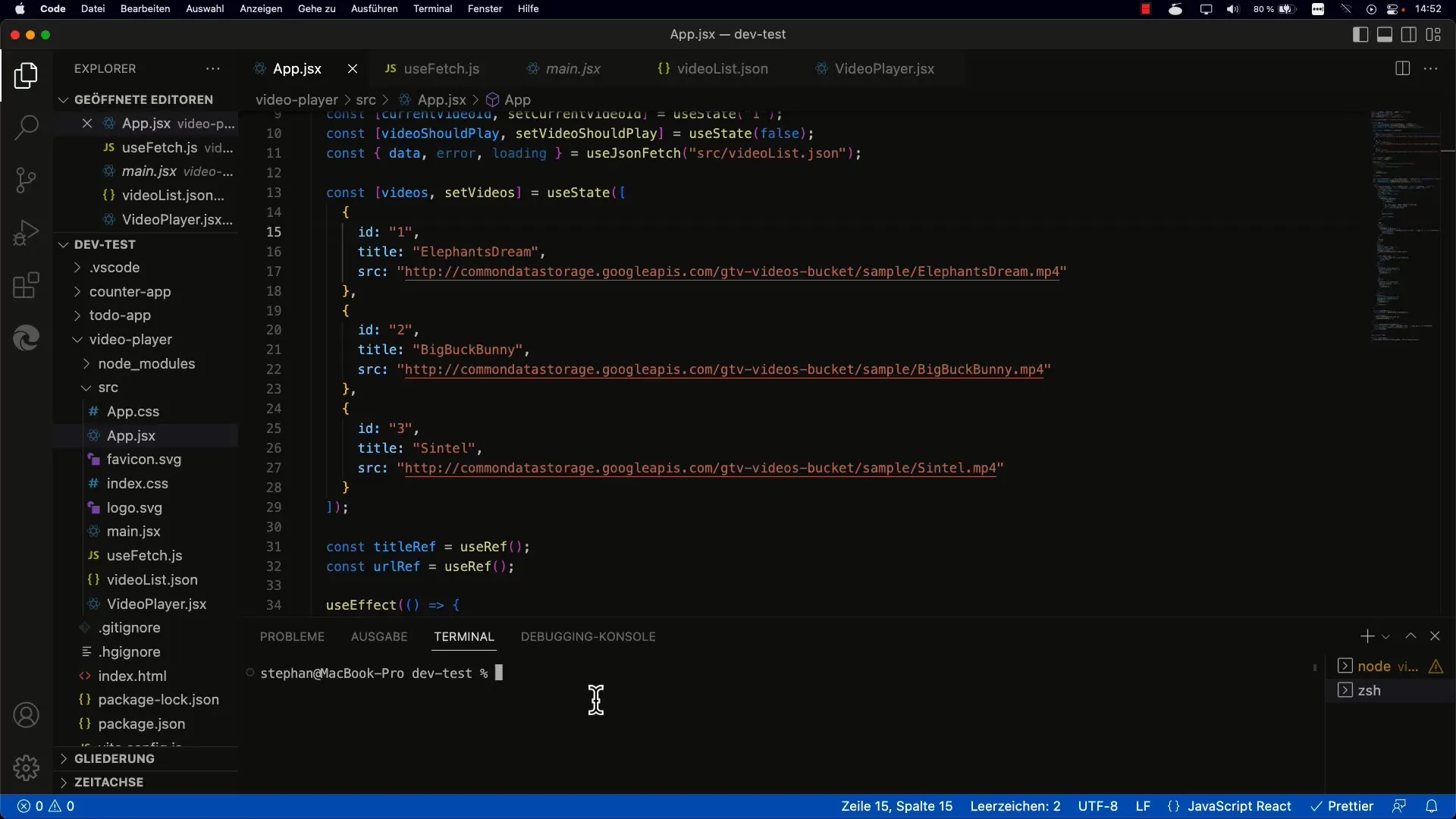
Using useImmer
Now that useImmer is installed, you can use it in your project. Simply import it into the file where you want to manage the state:
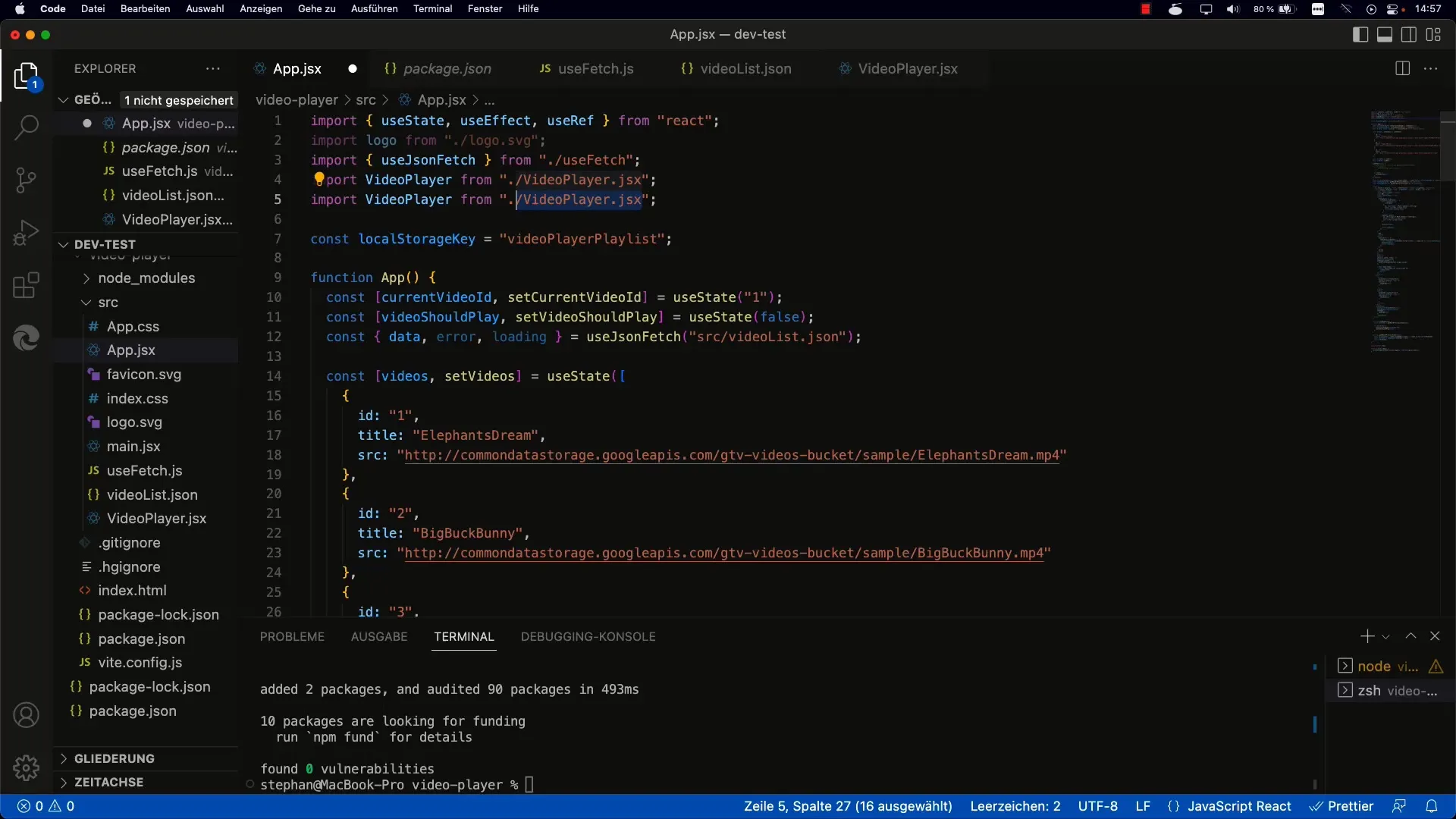
Getting Started with useImmer
Compared to useState, the structure of your code remains almost the same. Instead of useState, you use useImmer to get your current state and a function to change the state.
In this example, initialVideos is the initial value for your state. You can now use setVideos to make changes to your state.
Mutations with useImmer
With useImmer, you have the flexibility to mutate the state directly. This means that you can use methods like push or splice without having to manually create a new array or object. Here is an example of how you can add a new video:
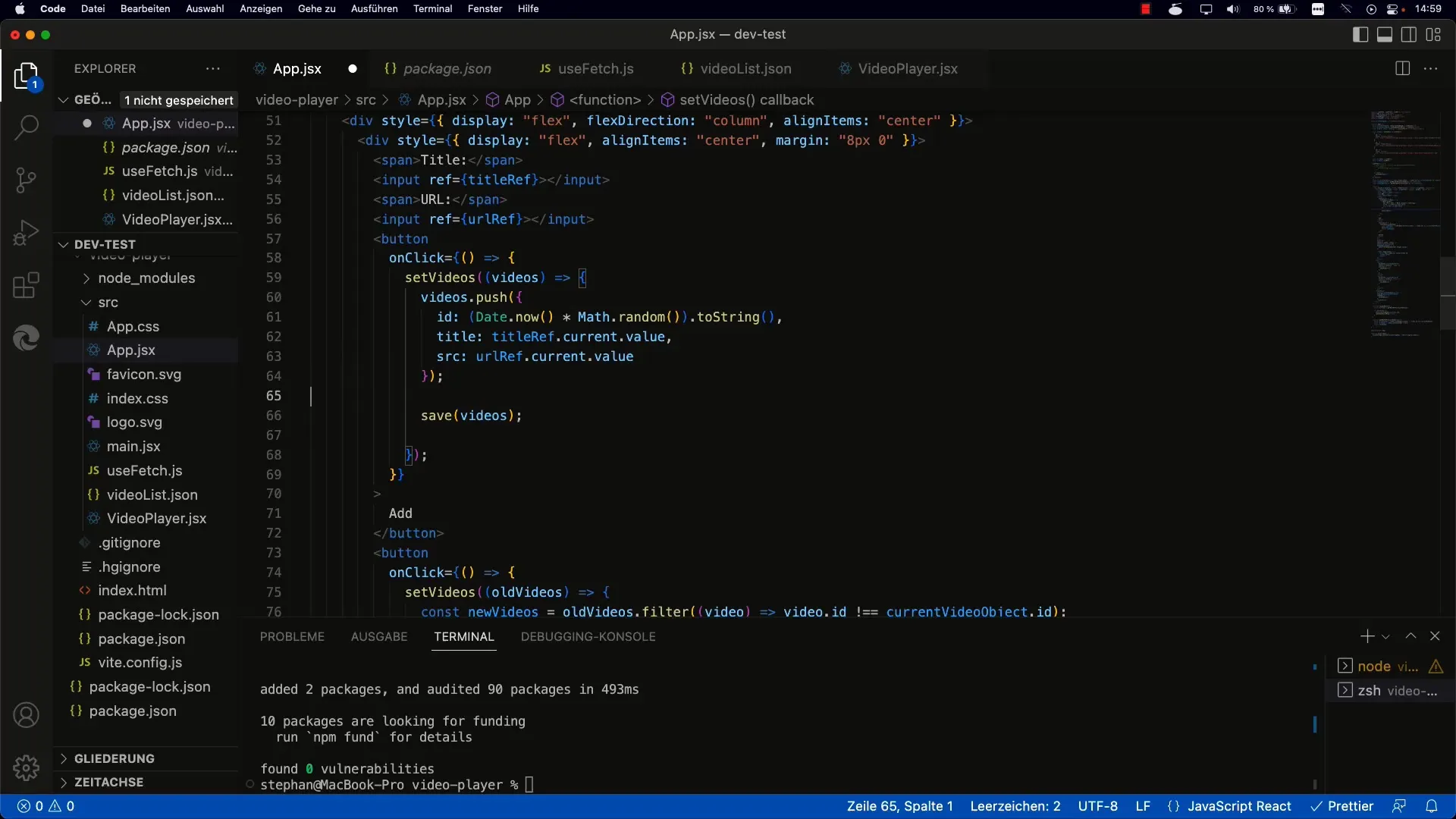
In this case, draft will be a mutable representation of your state. Any changes you make to it will automatically result in creating a new, immutable state.
Modifying Existing Entries
If you want to change an existing entry, it is also straightforward with useImmer. You simply need to find the entry in the array and make the desired changes:
Here you can access a specific video in the draft array and make the desired changes. These changes are automatically treated as new references.
Deleting an Entry
Deleting an entry is also easier. You can use filter or splice to remove the desired element. Here is an example using splice:
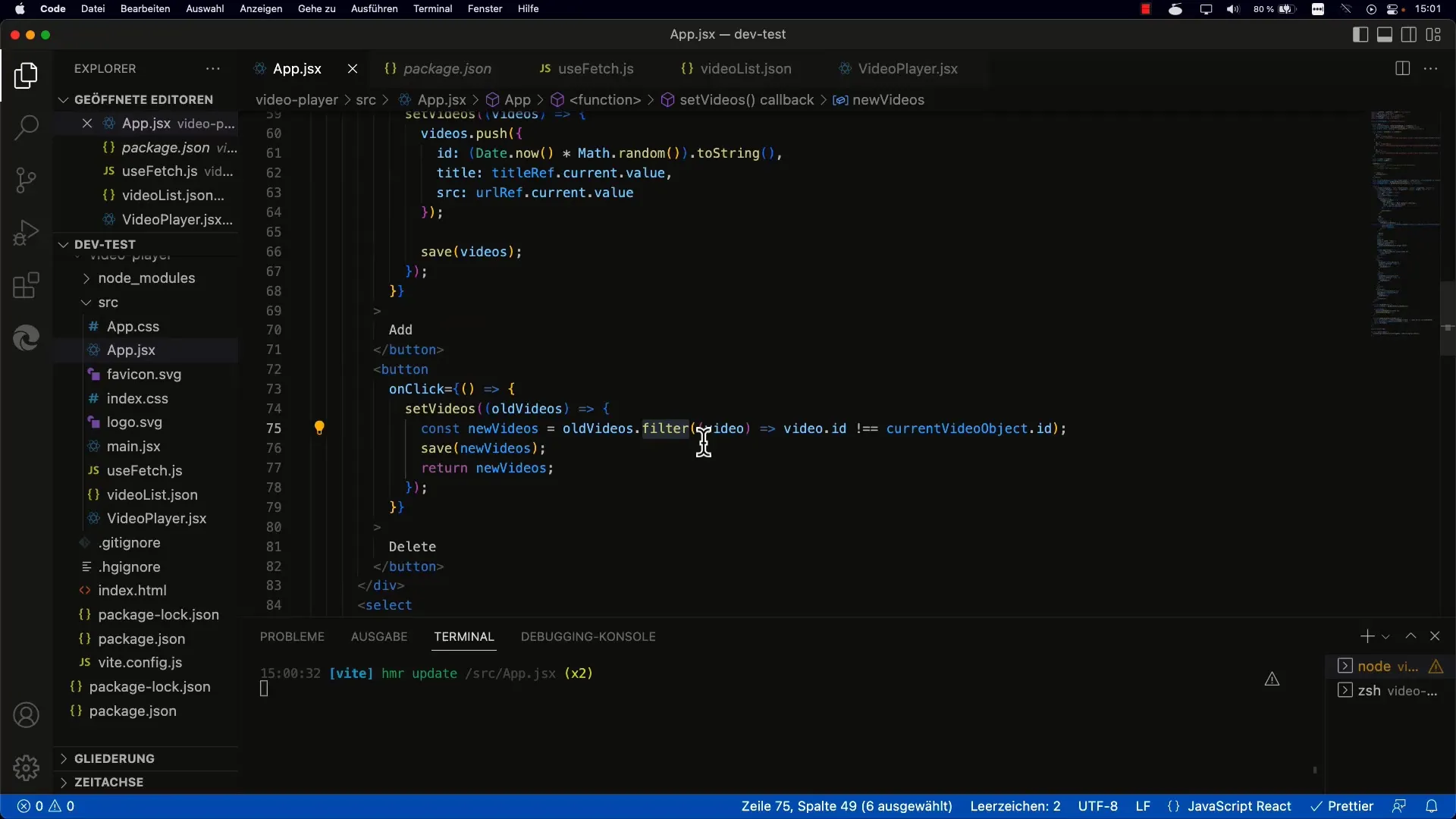
This removes the video with the corresponding ID from the draft array.
Integration into Existing Projects
Replacing useState with useImmer can significantly simplify and make your code more readable. You just need to make sure that wherever you have used useState, you are able to introduce useImmer.
It is important to note that using useImmer does not negatively affect performance. However, you should always ensure that your code remains clear and well-structured.
Summary
In this tutorial, you have learned how to use useImmer in your React project to simplify state management. You can now have direct access to mutations, making development more efficient and enjoyable. By automatically converting to immutable states, React retains control and ensures that all changes are properly recognized.
Frequently Asked Questions
What is useImmer?useImmer is a React Hook that allows you to mutably alter the state while retaining the benefits of immutability.
How do I install useImmer?You install useImmer with the command npm install immer.
How does adding an element with useImmer work?You can add a new element by using setVideos(draft => { draft.push(newVideo); });.
Can I modify existing entries with useImmer?Yes, you can modify existing entries by finding the corresponding entry in the draft array and making the desired changes.
What happens when I use useImmer in my project?With useImmer, you can directly perform mutations on your state, which cleans up and simplifies the code. A new immutable state is automatically created.