Efficient rendering is crucial when it comes to developing high-performance web applications. When dealing with extensive calculations within the rendering function, useMemo can be a helpful solution to utilize cached results for repeated renderings. In this guide, you will learn how useMemo works and how to implement it in your projects.
Key Takeaways
- useMemo helps optimize expensive calculations by storing their results while considering dependencies.
- It is important to use useMemo only for truly complex calculations to avoid unnecessary performance losses.
- Proper management of dependencies is crucial to keep the results up to date.
Step-by-Step Guide
Step 1: Basic Setup
Firstly, create a simple React component setup. For our example, we will use a toggle button and a checkbox to switch between two calculations: factorial calculation and summation.
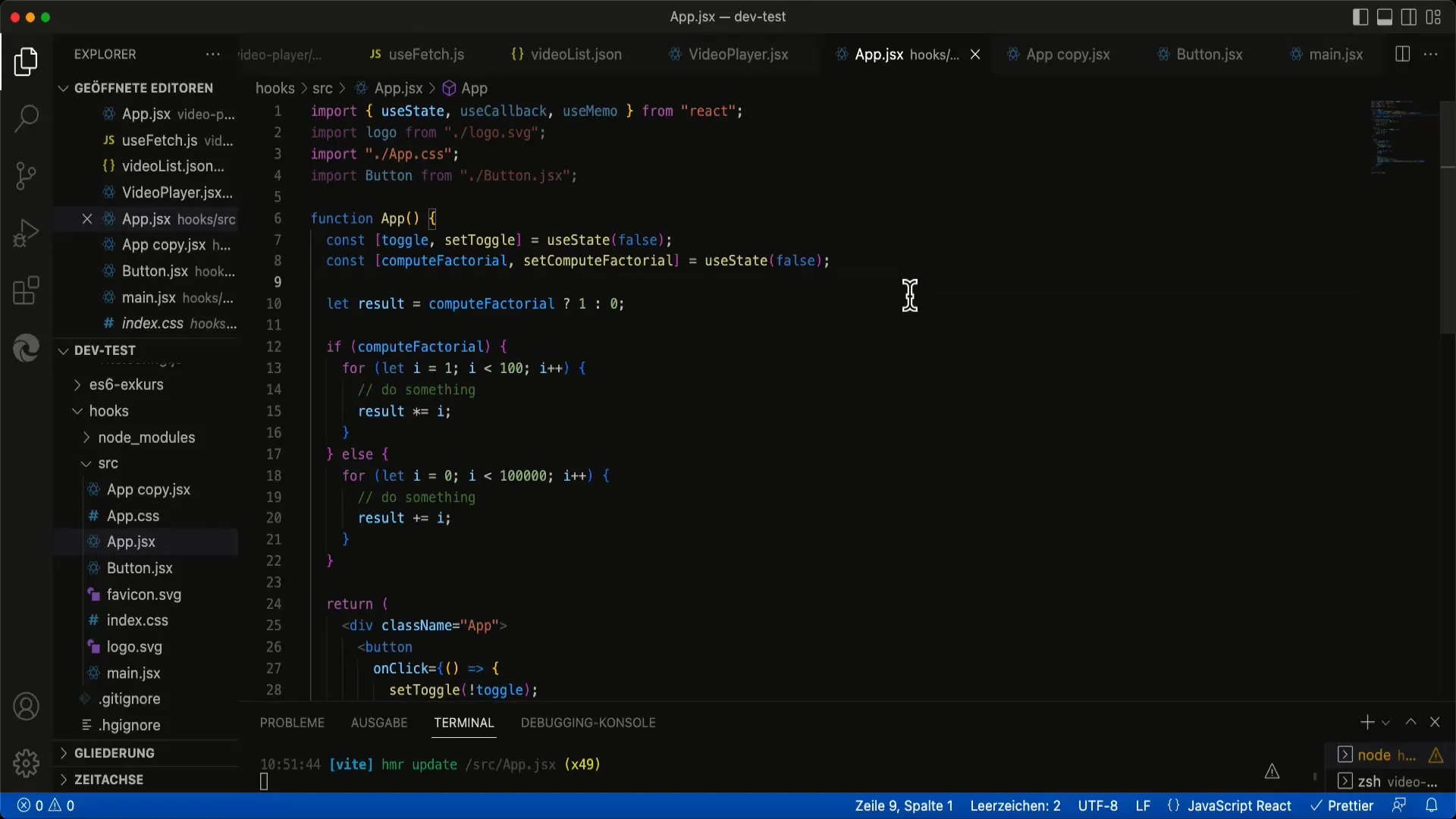
Step 2: Implement the Toggle Button
The toggle button switches between two states that control your program. A checkbox is added to decide whether to calculate the factorial or the sum.
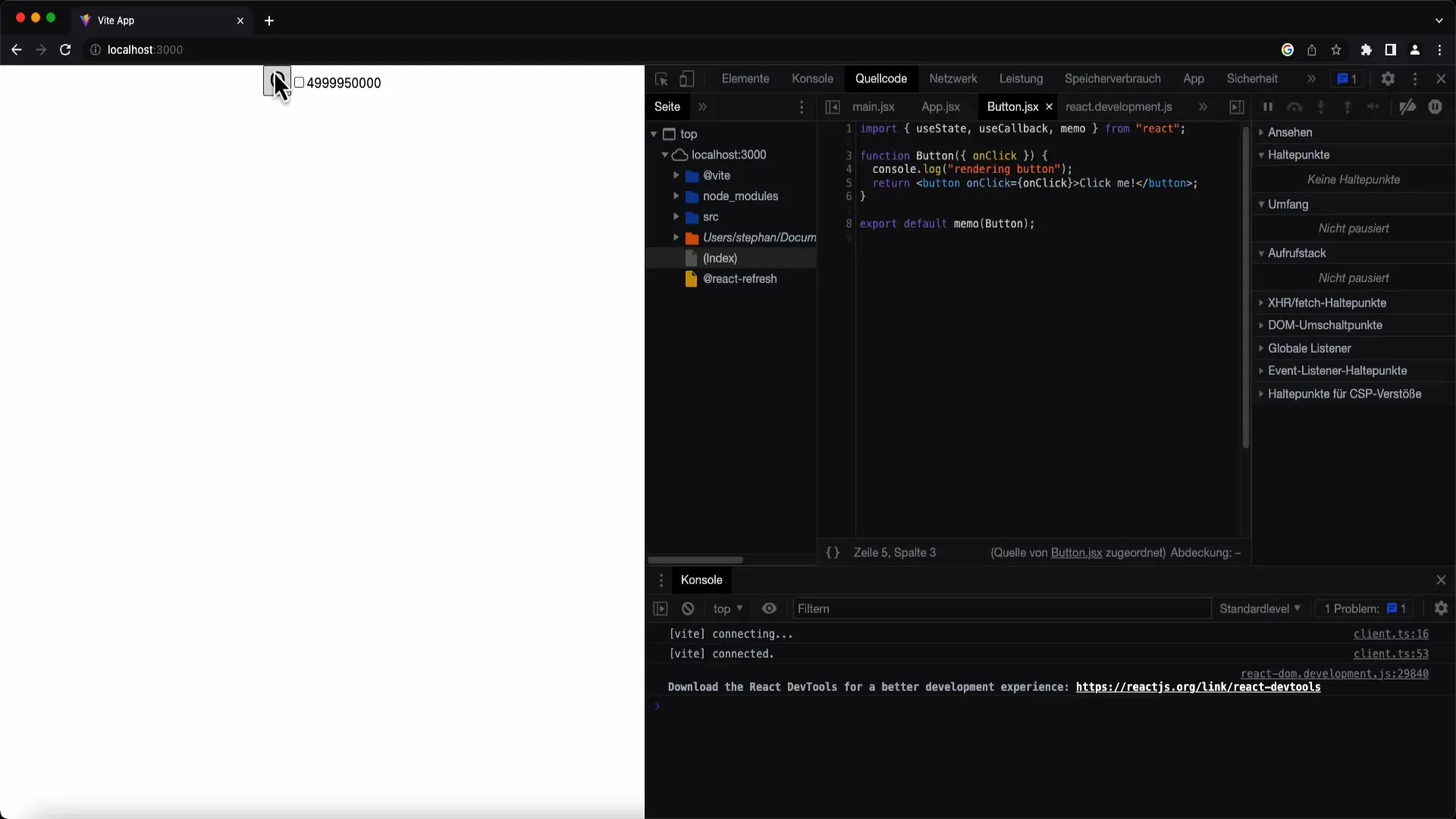
Step 3: Set Up State Management
Define the state for computeFactorial to determine if the factorial calculation should be active. If the checkbox is checked, computeFactorial is set to true; otherwise, it is set to false.
Step 4: Create the Calculation Functions
Create the functions that calculate the factorial and the sum. The code symbolizes that these are complex calculations. However, these functions will not yet be optimized with useMemo.
Step 5: Perform the Calculations
In the rendering process, you need to display the results of these calculations. In the example, the result is updated based on the state of computeFactorial.
Step 6: Introducing useMemo
Now, we will incorporate useMemo. Wrap the calculation functions in useMemo. This way, the function is called initially, and the result is cached.
Step 7: Manage Return Values
Ensure that the result of the calculation via useMemo is returned. This is done by utilizing the result of the function passed to useMemo.
Step 8: Define Dependencies
Add an array of dependencies as the second parameter. In this case, it is computeFactorial, which informs React when the function needs to be called again.
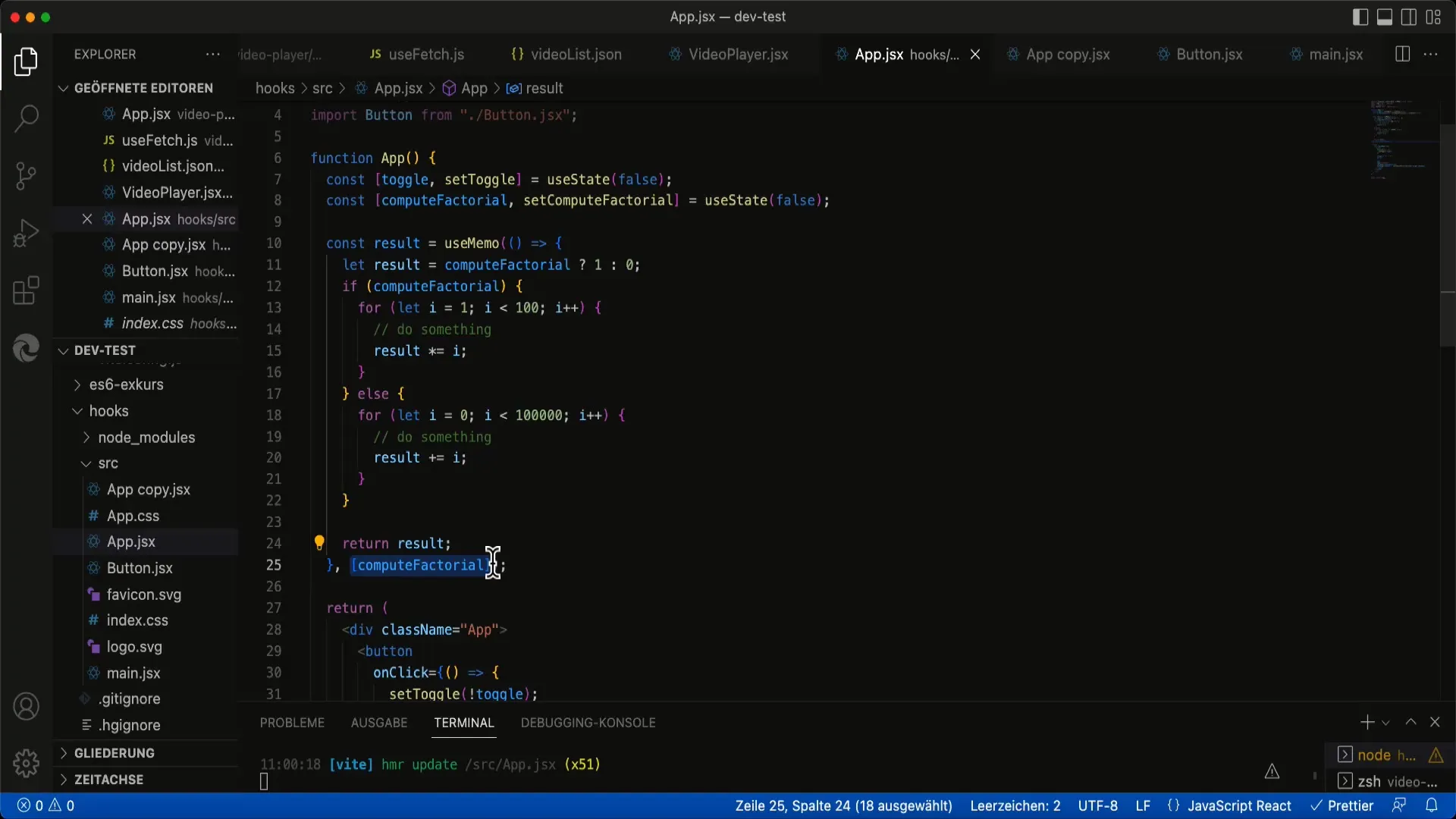
Step 9: Check the Optimization
To ensure everything is working, add a console command that indicates when the function is being called. Reload the component and test the functionality by switching between the toggle button and the checkbox.
Step 10: Analyze Results
Observe the console output: Pressing the toggle button should no longer trigger the complex calculation. The output demonstrates that useMemo effectively caches the results as long as the rendering dependencies do not change.
Summary
Using useMemo for optimizing render cycles in React can bring significant performance benefits, especially for complex calculations. Be sure to use useMemo responsibly to avoid excessive function calls during unnecessary rendering processes.
Frequently Asked Questions
What is the purpose of useMemo?useMemo stores the result of a function to avoid repeated and expensive calculations during rendering.
When should useMemo be used?useMemo should be used when expensive calculations occur in rendering functions, with the results heavily depending on specific dependencies.
What happens when dependencies change?When dependencies change, the stored function is called again, and the new result is cached.
Is useMemo always the best solution?Not necessarily. useMemo should only be used for complex calculations to avoid performance losses.##